아두이노 우노 R4 - 버튼 - 디바운스
Arduino Uno R4를 프로그래밍하여 버튼 누름 이벤트를 감지할 때, 한 번의 눌림이 여러 번 감지되는 경우가 있습니다. 이는 기계적인 요인으로 인해 버튼이나 스위치가 LOW와 HIGH 사이를 여러 번 빠르게 전환할 수 있기 때문입니다. 이를 "채터링"이라고 합니다. 채터링은 한 번의 버튼 눌림이 여러 번 눌린 것으로 감지되게 하여 일부 애플리케이션에서 오류를 발생시킬 수 있습니다. 이 튜토리얼은 이 문제를 해결하는 방법을 설명하며, 이는 버튼을 디바운싱하는 과정으로 알려져 있습니다.
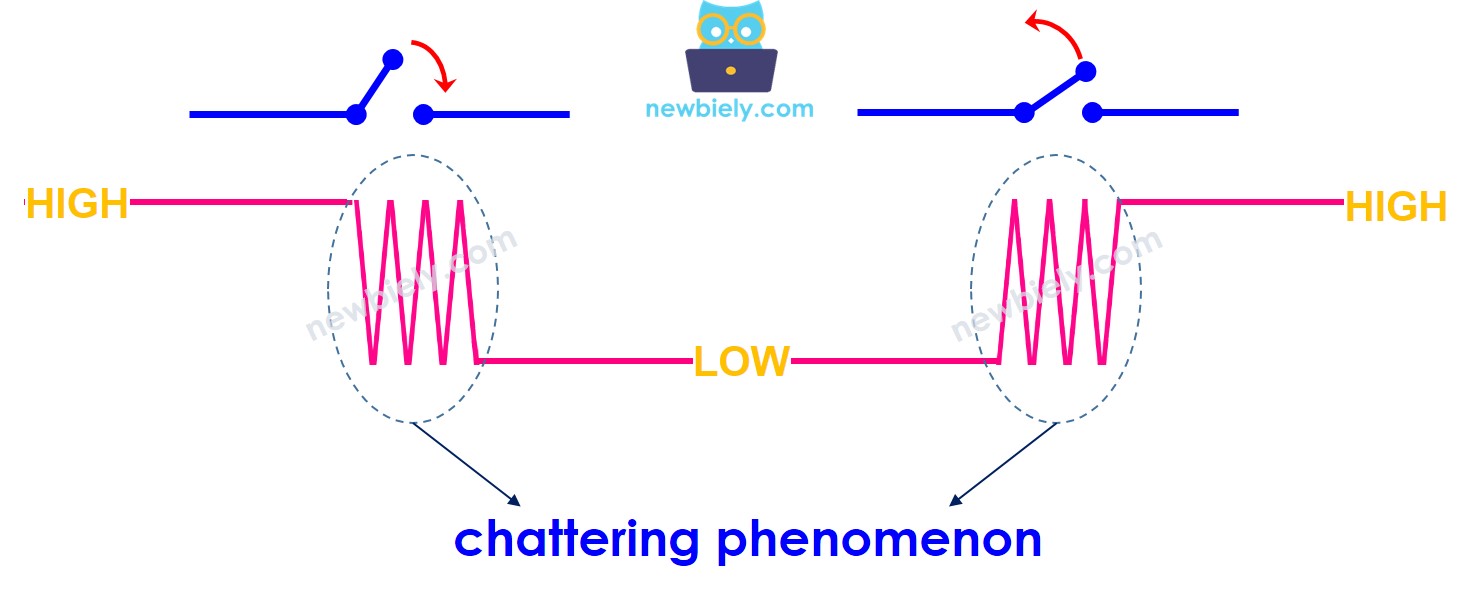
Hardware Preparation
1 | × | Arduino UNO R4 WiFi | Amazon | |
1 | × | Arduino UNO R4 Minima (Alternatively) | Amazon | |
1 | × | USB Cable Type-C | 쿠팡 | Amazon | |
1 | × | Breadboard-mount Button with Cap | 쿠팡 | Amazon | |
1 | × | Breadboard-mount Button Kit | 쿠팡 | Amazon | |
1 | × | Panel-mount Push Button | Amazon | |
1 | × | Breadboard | 쿠팡 | Amazon | |
1 | × | Jumper Wires | Amazon | |
1 | × | (Recommended) Screw Terminal Block Shield for Arduino UNO R4 | 쿠팡 | Amazon | |
1 | × | (Recommended) Breadboard Shield For Arduino UNO R4 | 쿠팡 | Amazon | |
1 | × | (Recommended) Enclosure For Arduino UNO R4 | Amazon | |
1 | × | (Recommended) Power Splitter For Arduino UNO R4 | Amazon |
버튼 정보
해당 튜토리얼에서 버튼에 대해 알아보세요(핀아웃, 작동, 프로그래밍). 버튼에 익숙하지 않다면 다음 내용을 참고하세요:
Wiring Diagram
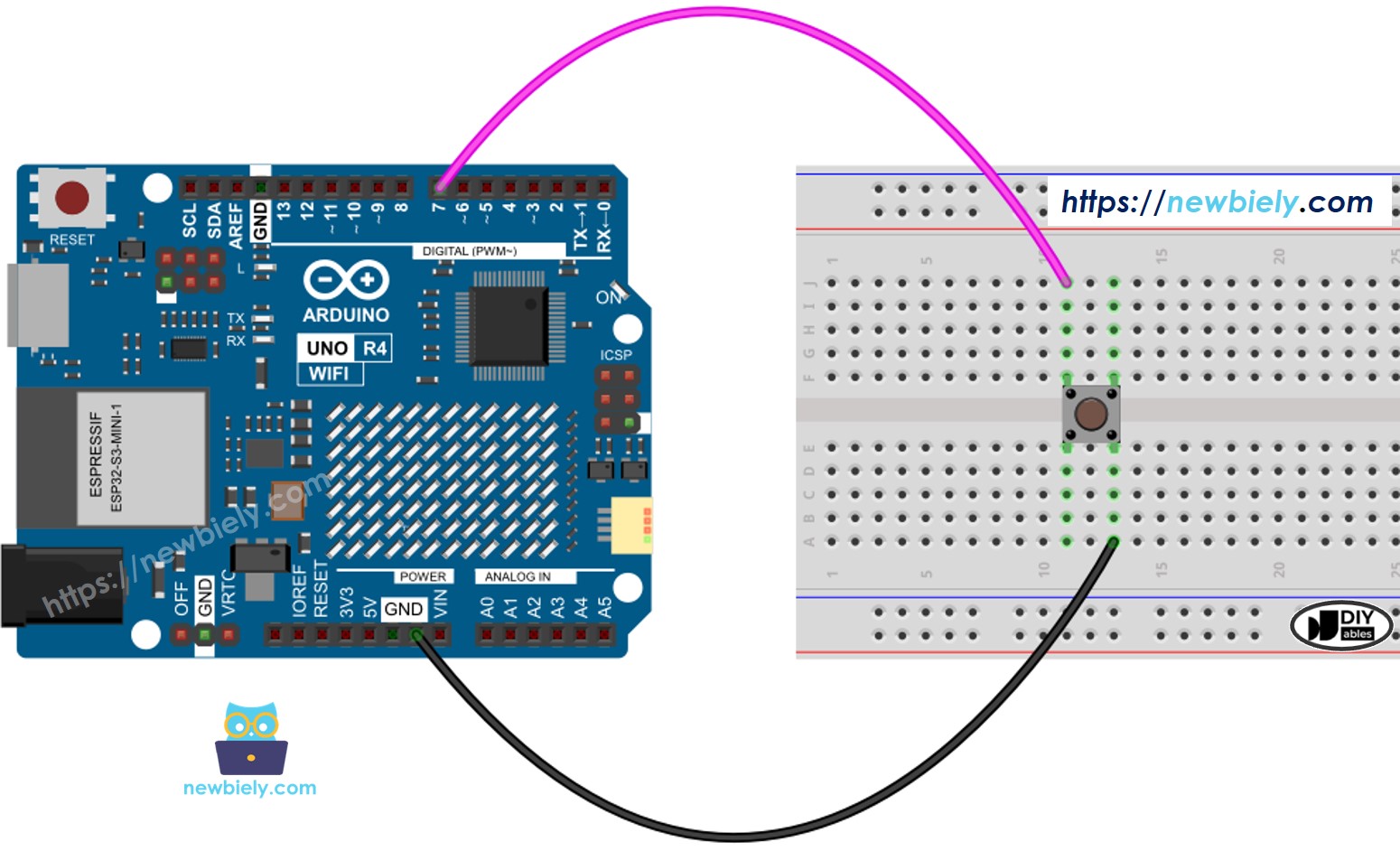
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
Arduino UNO R4 코드에서 디바운스 없이와 함께하는 경우를 살펴보고 비교하여 그 동작을 관찰해 봅시다.
Arduino Uno R4 - 디바운스 없는 버튼
디바운스를 배우기 전에, 디바운스를 사용하지 않은 코드를 보고 그 동작을 살펴봅시다.
Detailed Instructions
다음 지침을 단계별로 따르세요:
- Arduino Uno R4 WiFi/Minima를 처음 사용하는 경우, Arduino IDE에서 Arduino Uno R4 WiFi/Minima 환경 설정하기 튜토리얼을 참조하세요.
- 제공된 다이어그램에 따라 부품을 연결하세요.
- USB 케이블을 사용하여 Arduino Uno R4 보드를 컴퓨터에 연결하세요.
- 컴퓨터에서 Arduino IDE를 실행하세요.
- 적절한 Arduino Uno R4 보드(예: Arduino Uno R4 WiFi)와 COM 포트를 선택하세요.
- 위 코드를 복사하여 Arduino IDE에 열어보세요.
- Arduino IDE에서 코드를 Arduino UNO R4로 전송하려면 Upload 버튼을 클릭하세요.
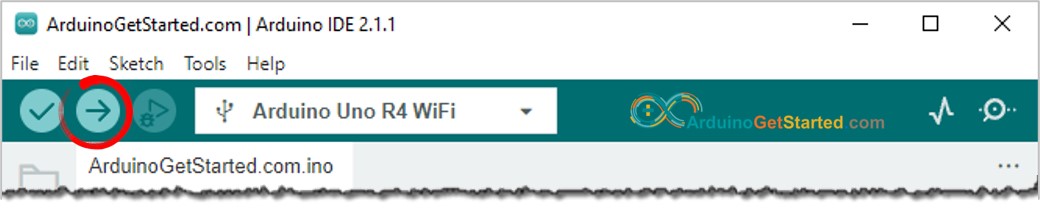
- 시리얼 모니터를 여세요.
- 버튼을 몇 초 동안 눌렀다가 놓으세요.
- 결과를 시리얼 모니터에서 확인하세요.
보시다시피 버튼을 한 번만 눌렀다 놓았습니다. 그러나 아두이노는 이를 여러 번의 눌림과 릴리스로 인식합니다.
※ NOTE THAT:
DEBOUNCE_TIME의 값은 다양한 애플리케이션에 따라 달라집니다. 각 애플리케이션은 고유한 값을 사용할 수 있습니다.
아두이노 우노 R4 - 디바운스 버튼
Detailed Instructions
- 위의 코드를 복사하여 Arduino IDE로 엽니다.
- Arduino IDE에서 Upload 버튼을 눌러 코드를 Arduino UNO R4에 전송합니다.
- 시리얼 모니터를 엽니다.
- 버튼을 몇 초간 누른 상태에서 손을 뗍니다.
- 시리얼 모니터를 확인합니다.
보시다시피, 버튼을 한 번 눌렀다가 놓았습니다. Arduino는 이를 올바르게 하나의 누름과 놓음으로 감지하여 채터링을 제거합니다.
우리는 간단하게 만들었습니다: 라이브러리를 사용한 Arduino UNO R4 버튼 디바운스 코드
버튼을 많이 사용하는 초보자들을 위해 ezButton이라는 라이브러리를 만들어 더 간단한 방법을 제공했습니다. ezButton 라이브러리에 대한 자세한 내용은 여기에서 확인할 수 있습니다.
몇 가지 예제 코드를 봅시다.
아두이노 UNO R4 단일 버튼 디바운스 코드
아두이노 UNO R4 다중 버튼 바운스 방지 코드
세 개의 버튼에 대해 디바운싱을 해봅시다. 아래는 Arduino UNO R4와 세 개의 버튼 간의 배선도입니다:
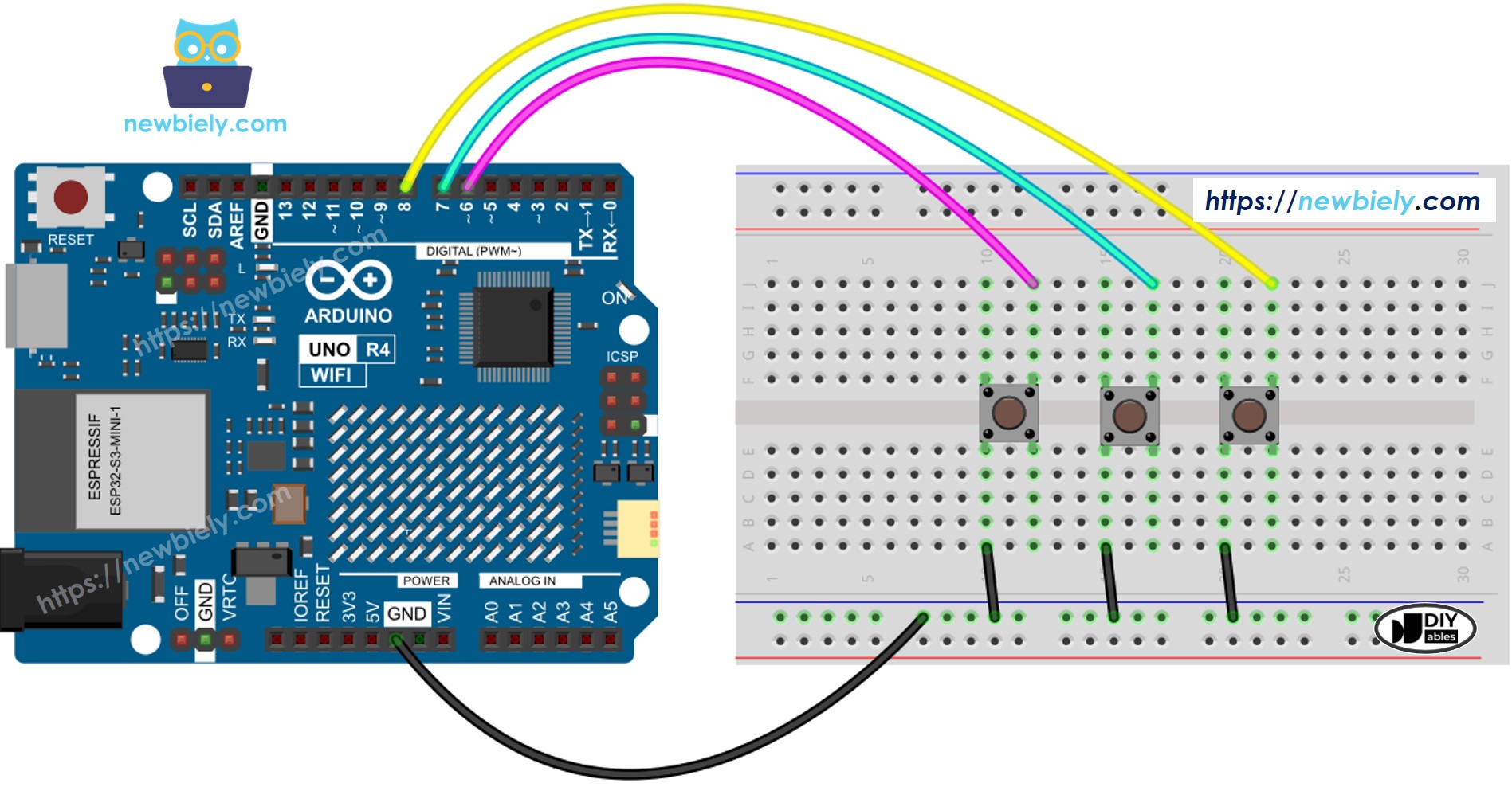
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
Video Tutorial
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.