아두이노 우노 R4 온도 센서 OLED
이 가이드에서는 Arduino UNO R4을(를) 프로그래밍하여 DS18B20 센서에서 온도를 읽고 OLED 화면에 표시하는 방법을 배울 것입니다.
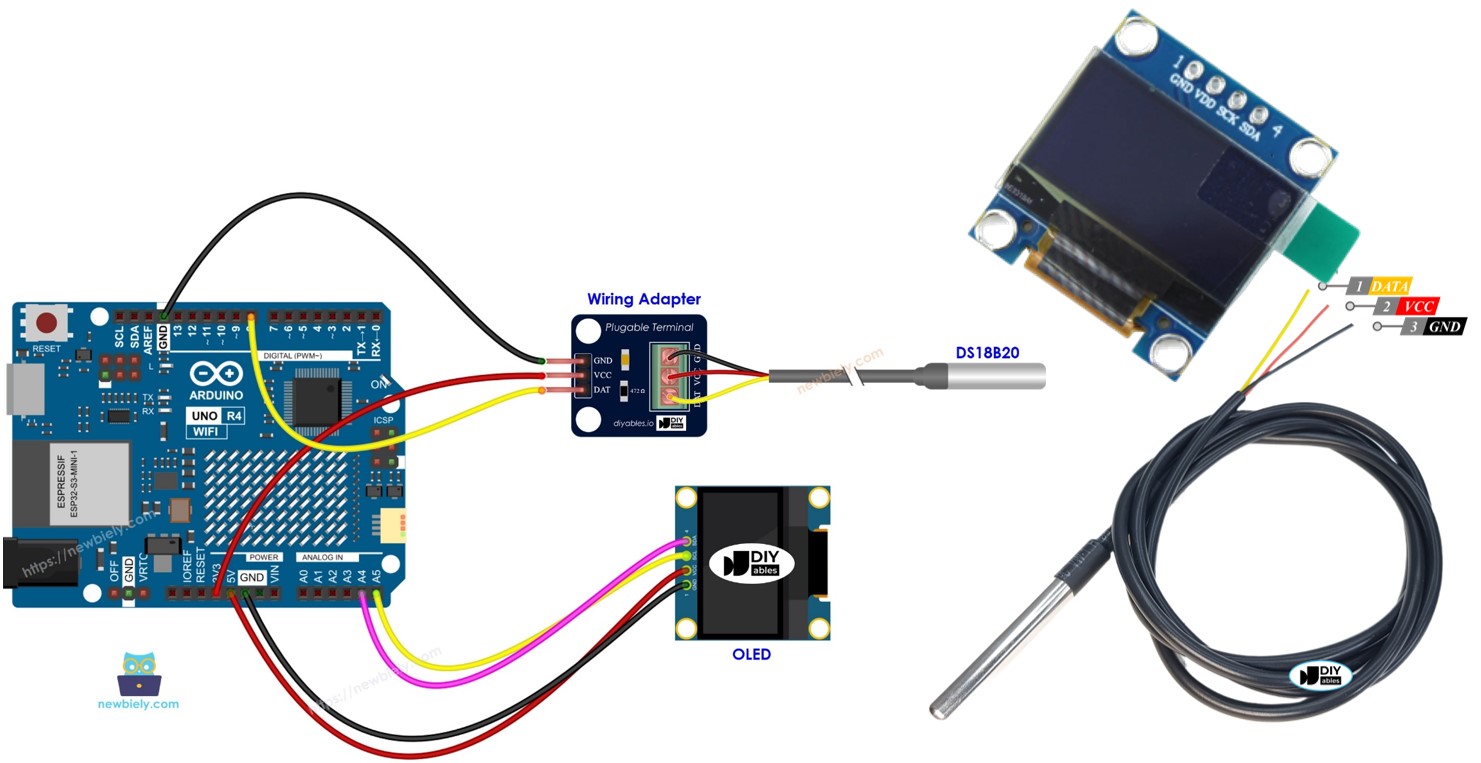
준비물
1 | × | 아두이노 우노 R4 와이파이 | 쿠팡 | 아마존 | |
1 | × | (또는) 아두이노 우노 R4 미니마 | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-A to 타입-C (USB-A PC용) | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-C to 타입-C (USB-C PC용) | 아마존 | |
1 | × | SSD1306 I2C OLED 디스플레이 128x64 | 쿠팡 | 아마존 | |
1 | × | SSD1306 I2C OLED 디스플레이 128x32 | 아마존 | |
1 | × | DS18B20 온도 센서 (어댑터 포함) | 쿠팡 | 아마존 | |
1 | × | DS18B20 온도 센서(어댑터 없음) | 아마존 | |
1 | × | 브레드보드 | 쿠팡 | 아마존 | |
1 | × | 점퍼케이블 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 스크루 터미널 블록 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 브레드보드 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 케이스 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 전원 분배기 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 프로토타이핑 베이스 플레이트 & 브레드보드 키트 | 아마존 |
공개: 이 포스팅 에 제공된 일부 링크는 아마존 제휴 링크입니다. 이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다.
OLED 및 DS18B20 온도 센서에 대하여
이 튜토리얼에서 OLED 및 DS18B20 온도 센서(핀 배열, 기능 및 프로그래밍 포함)에 대해 알아보세요.
선연결
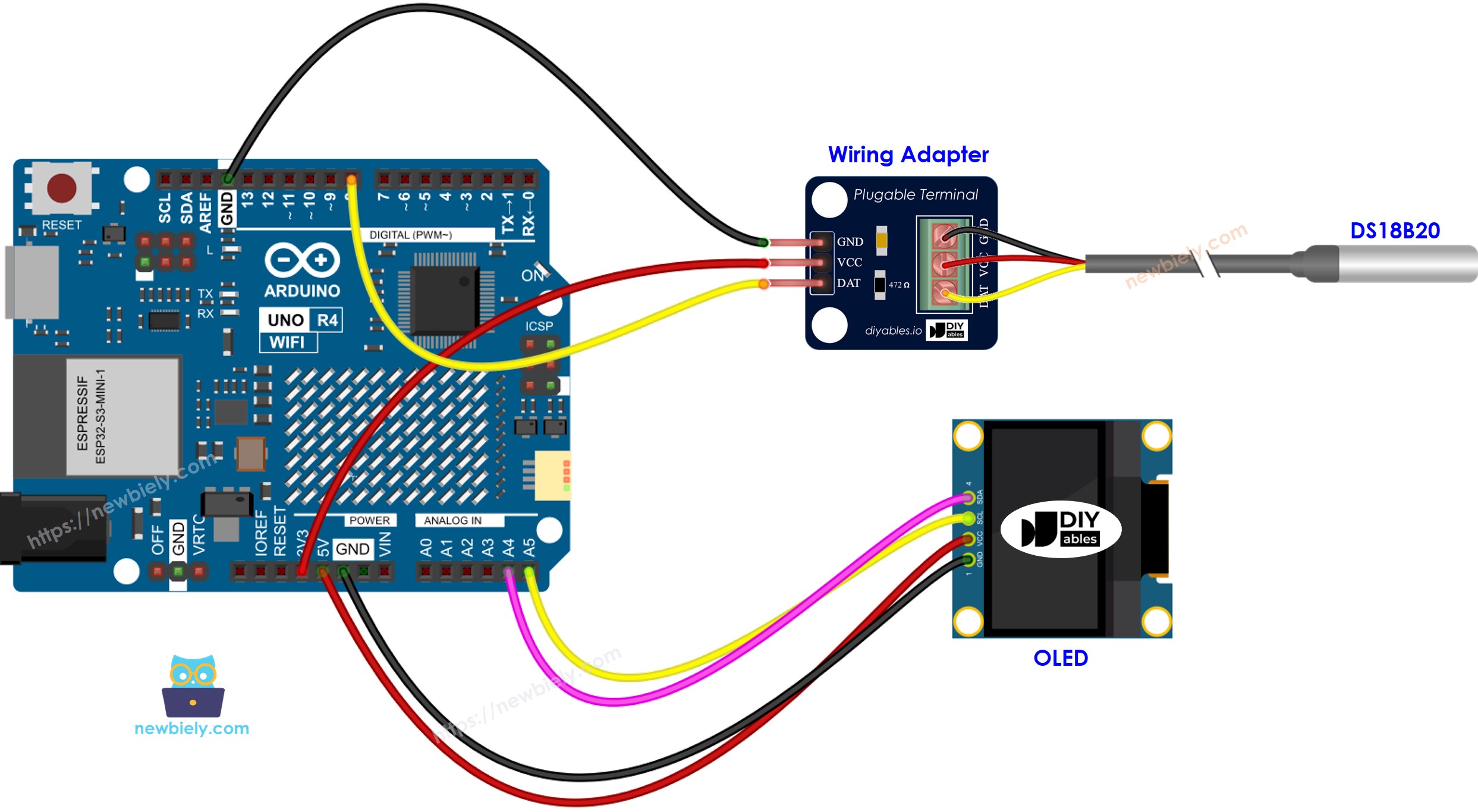
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
Arduino Uno R4와 기타 부품에 전원을 공급하는 가장 효과적인 방법을 확인하시려면, 아래 링크를 참조하세요: 아두이노 우노 R4 전원 공급 방법.
간단한 설치를 위해 DS18B20 센서와 배선 어댑터를 구매할 것을 권장합니다. 어댑터에는 저항이 포함되어 있어 추가 저항이 필요하지 않습니다.
Arduino UNO R4 코드 - DS18B20 온도 센서에서 온도를 측정하고 OLED에 표시하기
/*
* 이 아두이노 우노 R4 코드는 newbiely.kr 에서 개발되었습니다
* 이 아두이노 우노 R4 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino-uno-r4/arduino-uno-r4-temperature-sensor-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#define OLED_WIDTH 128 // OLED display width, in pixels
#define OLED_HEIGHT 64 // OLED display height, in pixels
#define SENSOR_PIN 8 // The Arduino UNO R4 pin connected to DS18B20 sensor
Adafruit_SSD1306 oled(OLED_WIDTH, OLED_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
OneWire oneWire(SENSOR_PIN); // setup a oneWire instance
DallasTemperature DS18B20(&oneWire); // pass oneWire to DallasTemperature library
String temperature_str;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
DS18B20.begin(); // initialize the sensor
temperature_str.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
DS18B20.requestTemperatures(); // send the command to get temperatures
float temperature_C = DS18B20.getTempCByIndex(0); // read temperature in Celsius
temperature_str = String(temperature_C, 2); // two decimal places
temperature_str += char(247) + String("C");
Serial.println(temperature_str); // print the temperature in Celsius to Serial Monitor
oled_display_center(temperature_str);
}
void oled_display_center(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// center the display both horizontally and vertically
oled.clearDisplay(); // clear display
oled.setCursor((OLED_WIDTH - width) / 2, (OLED_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
자세한 사용 방법
다음 지침을 단계별로 따르십시오:
- Arduino Uno R4 WiFi/Minima를 처음 사용하는 경우, 아두이노 우노 R4 - 소프트웨어 설치을 참조하세요.
- 제공된 다이어그램에 따라 OLED와 온도 센서를 Arduino Uno R4 보드에 연결하세요.
- USB 케이블을 사용하여 Arduino Uno R4 보드를 컴퓨터에 연결하세요.
- 컴퓨터에서 Arduino IDE를 실행하세요.
- 적절한 Arduino Uno R4 보드(e.g., Arduino Uno R4 WiFi) 및 COM 포트를 선택하세요.
- Arduino IDE의 왼쪽에 있는 Libraries 아이콘을 클릭하세요.
- 검색 상자에 “SSD1306”을 입력한 다음 Adafruit의 SSD1306 라이브러리를 찾으세요.
- 라이브러리를 추가하려면 “설치” 버튼을 누르세요.
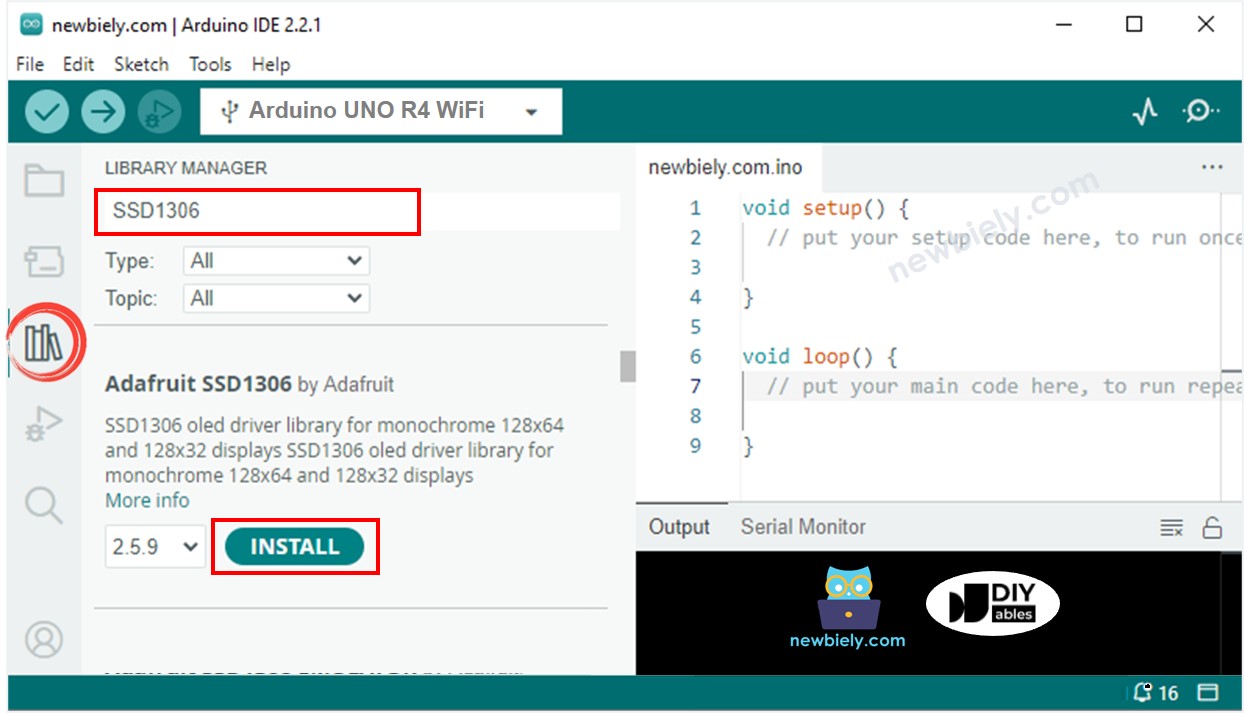
- 추가 라이브러리를 설치해야 합니다.
- 필요한 모든 라이브러리를 설치하려면 Install All 버튼을 클릭하세요.
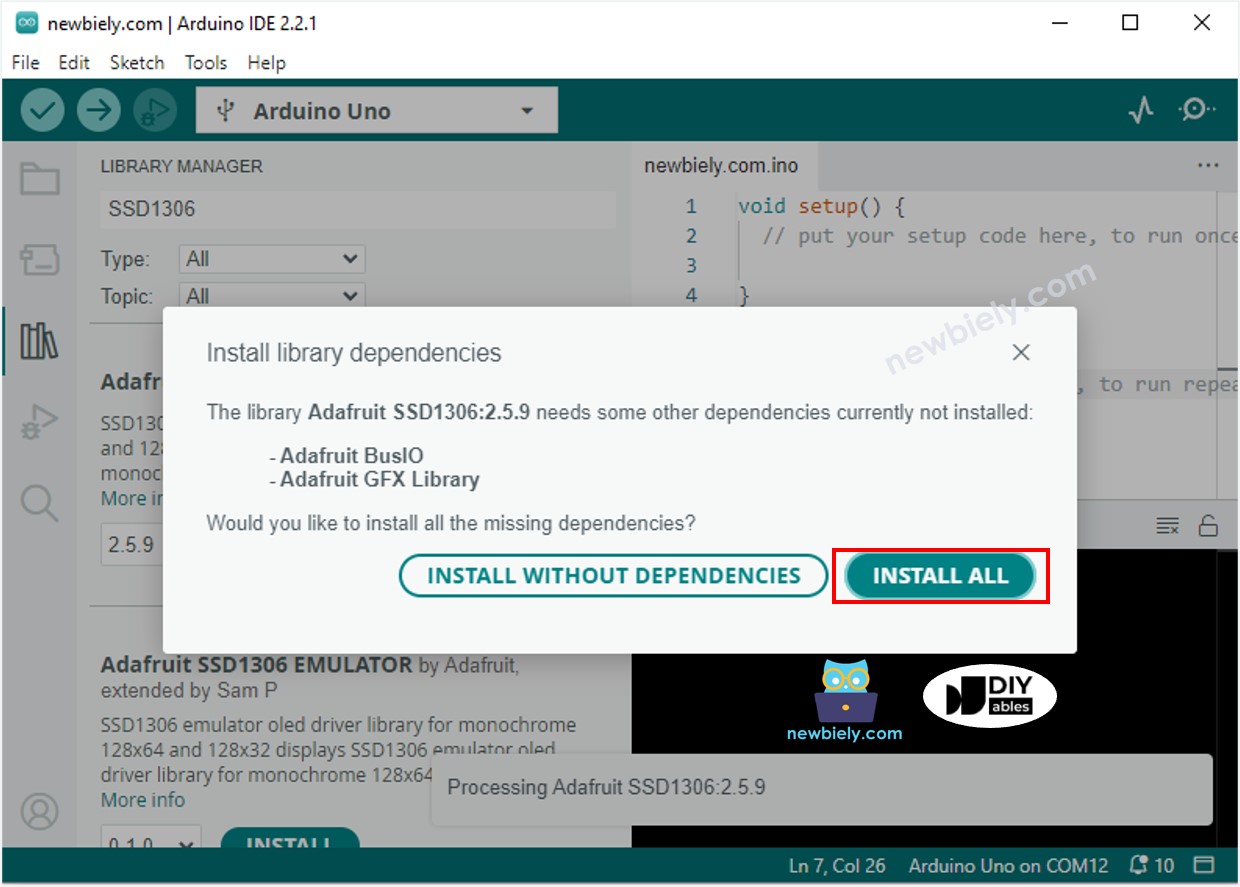
- 검색 상자에 "DallasTemperature"를 입력하고 Miles Burton의 DallasTemperature 라이브러리를 찾습니다.
- DallasTemperature 라이브러리를 설치하려면 Install 버튼을 누르세요.
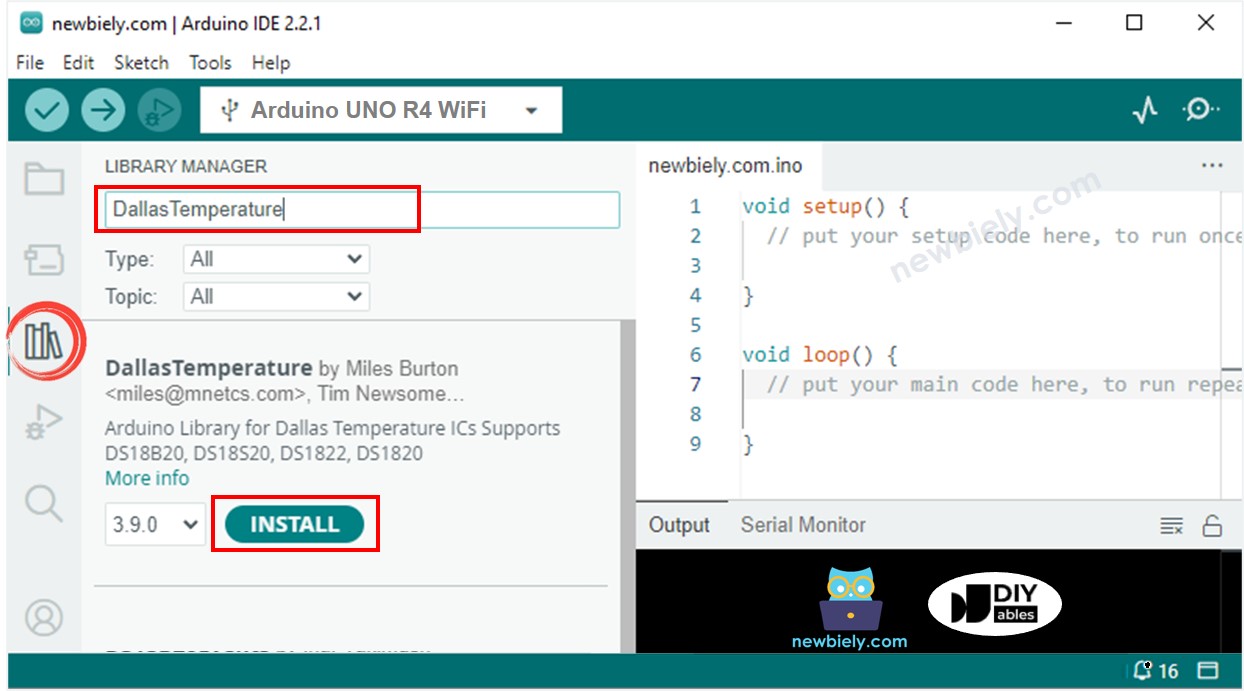
- 라이브러리 종속성을 설치해야 합니다.
- Install All 버튼을 클릭하여 OneWire 라이브러리를 설치합니다.
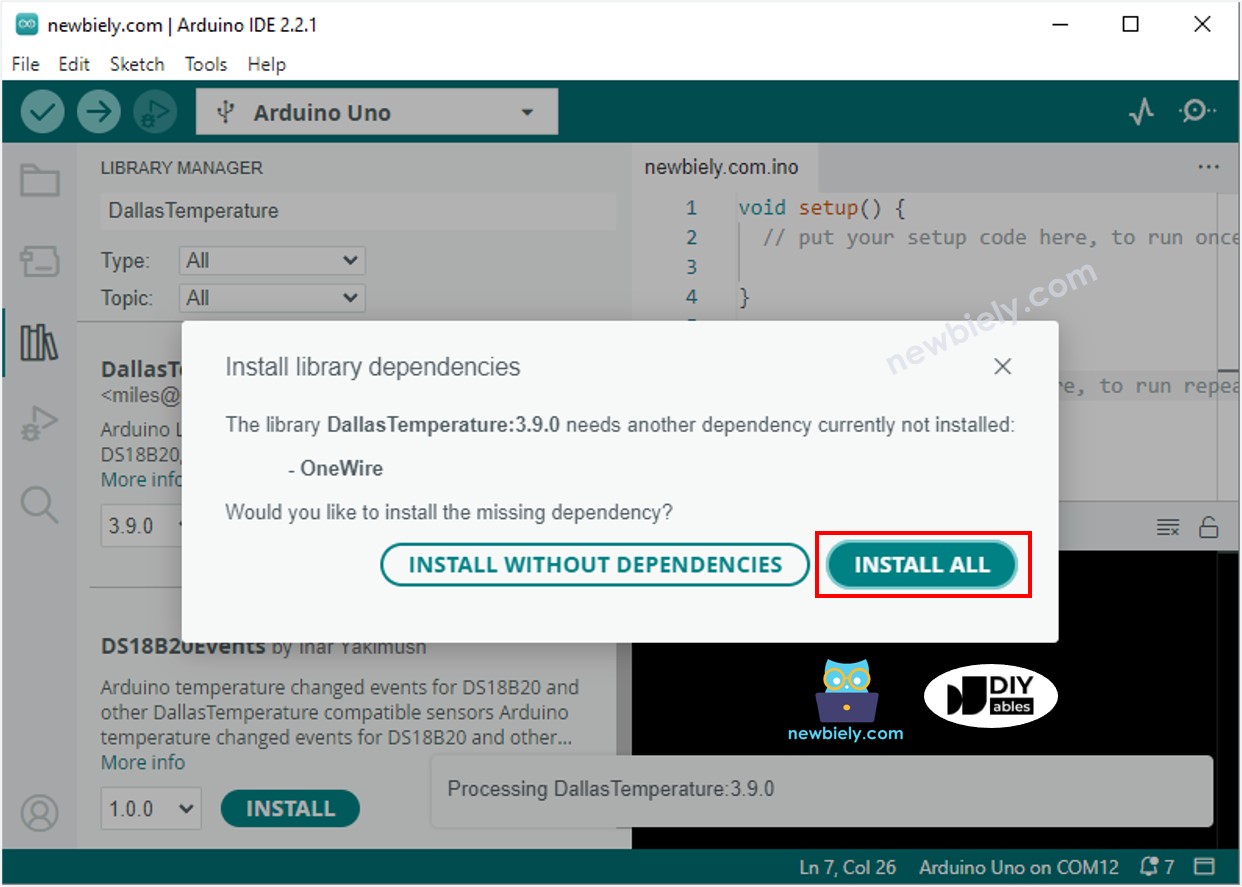
- 위의 코드를 복사하여 Arduino IDE에서 엽니다
- Arduino IDE에서 Upload 버튼을 눌러 코드를 Arduino UNO R4로 전송합니다
- 센서를 뜨거운 물이나 차가운 물에 넣거나 손에 잡습니다
- OLED에 표시되는 결과를 확인합니다
※ 주의:
코드는 OLED 디스플레이에서 텍스트를 가로 및 세로로 자동으로 중앙에 배치합니다.
동영상
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.