아두이노 우노 R4 DHT11 OLED
이 가이드에서는 DHT11 모듈을 사용하여 온도와 습도를 측정하고 OLED 화면에 표시하는 방법을 배웁니다.
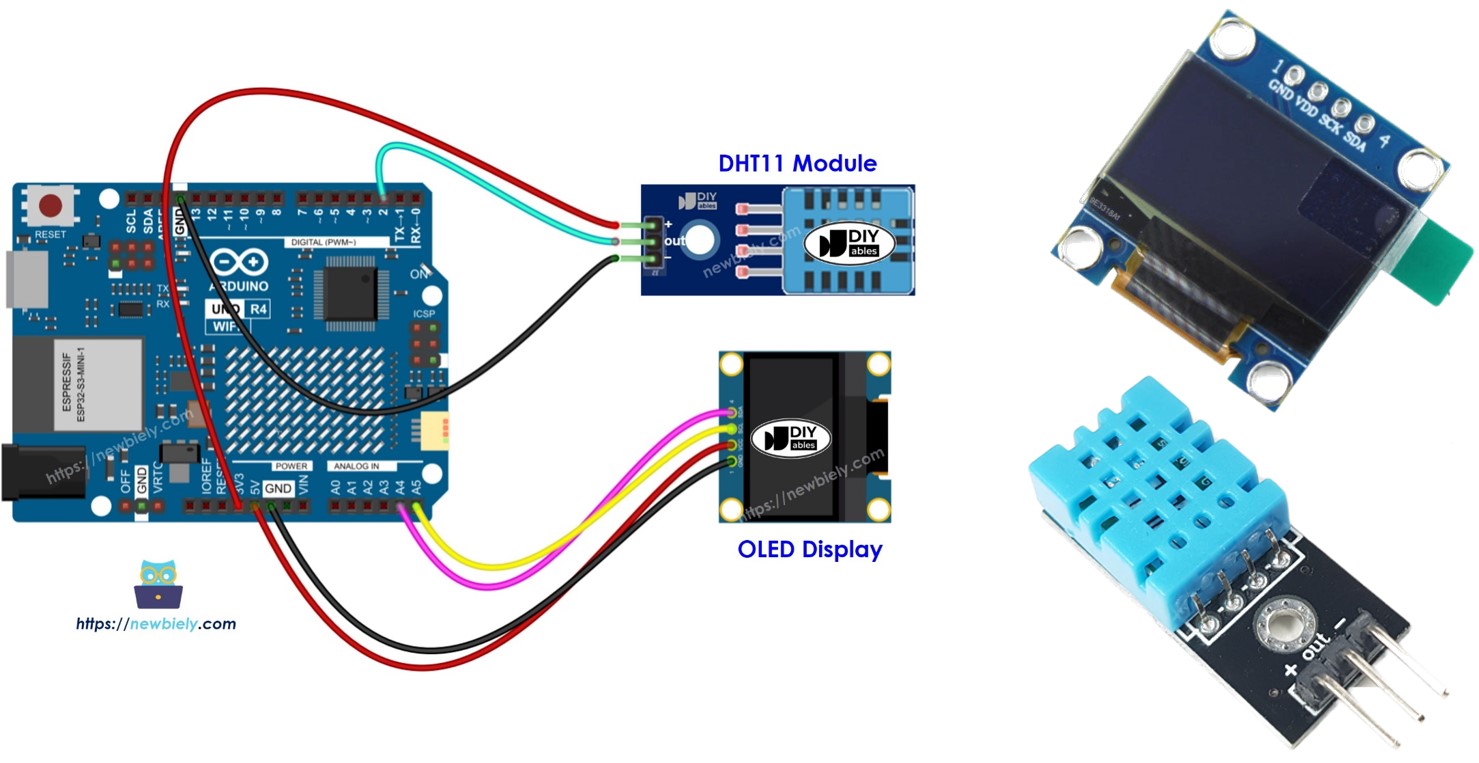
준비물
1 | × | 아두이노 우노 R4 와이파이 | 쿠팡 | 아마존 | |
1 | × | (또는) 아두이노 우노 R4 미니마 | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-A to 타입-C (USB-A PC용) | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-C to 타입-C (USB-C PC용) | 아마존 | |
1 | × | SSD1306 I2C OLED 디스플레이 128x64 | 쿠팡 | 아마존 | |
1 | × | SSD1306 I2C OLED 디스플레이 128x32 | 아마존 | |
1 | × | DHT11 온도 습도 센서 모듈 | 아마존 | |
1 | × | 점퍼케이블 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 스크루 터미널 블록 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 브레드보드 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 케이스 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노 R4용 전원 분배기 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 프로토타이핑 베이스 플레이트 & 브레드보드 키트 | 아마존 |
공개: 이 포스팅 에 제공된 일부 링크는 아마존 제휴 링크입니다. 이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다.
OLED 디스플레이, DHT11 온습도 센서 소개
아래의 튜토리얼에서 OLED 디스플레이와 DHT11 온도 습도 센서 (설치, 기능, 프로그래밍)에 대해 배워보세요:
- 아두이노 우노 R4 - OLED 튜토리얼
- 아두이노 우노 R4 - DHT11 튜토리얼
선연결
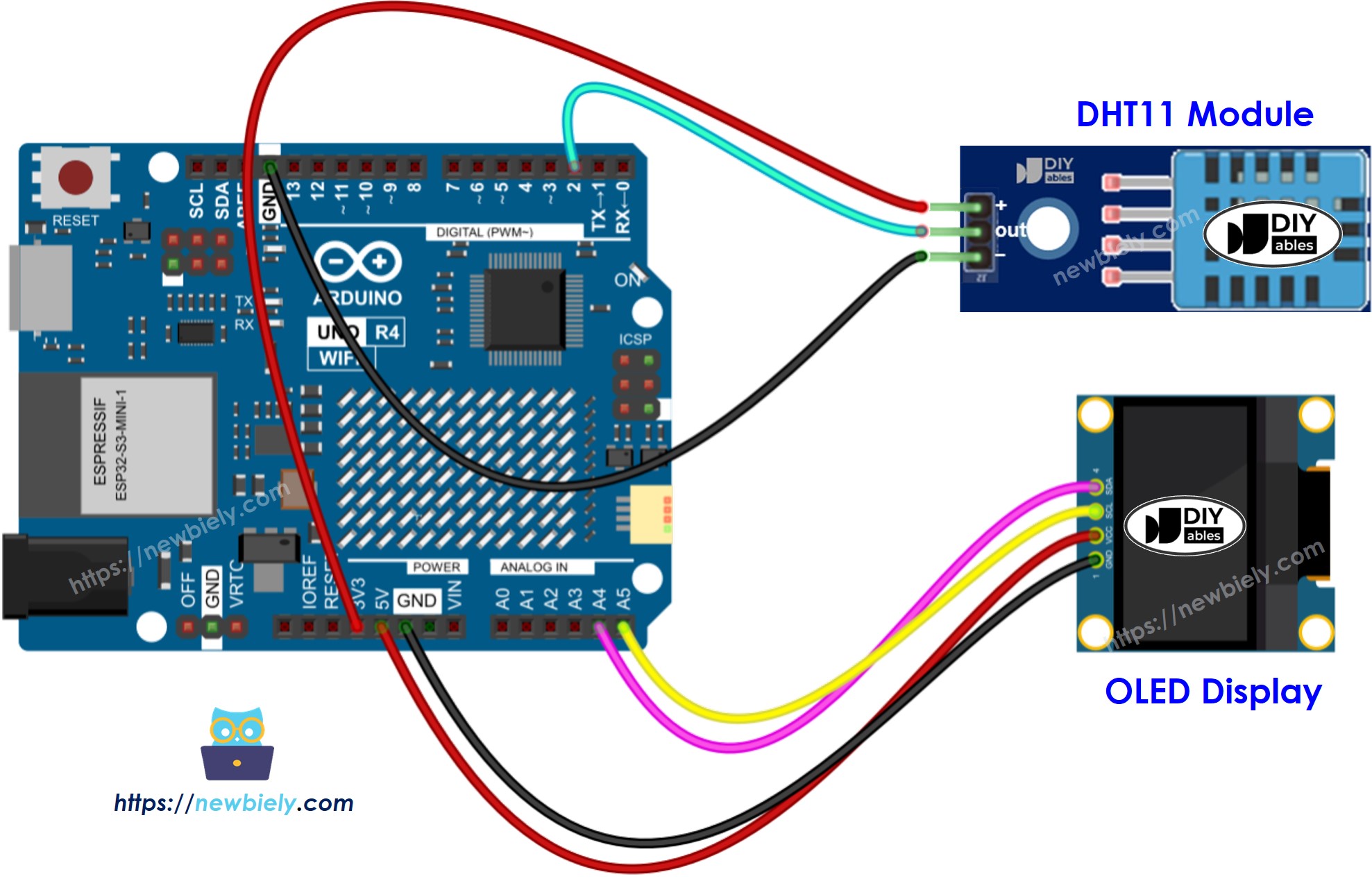
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
Arduino Uno R4와 기타 부품에 전원을 공급하는 가장 효과적인 방법을 확인하시려면, 아래 링크를 참조하세요: 아두이노 우노 R4 전원 공급 방법.
Arduino UNO R4 코드 - DHT11 센서 - OLED
/*
* 이 아두이노 우노 R4 코드는 newbiely.kr 에서 개발되었습니다
* 이 아두이노 우노 R4 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino-uno-r4/arduino-uno-r4-dht11-temperature-humidity-sensor-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <DHT.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define DHT11_PIN 2 // The Arduino UNO R4 pin connected to DHT11 sensor
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
DHT dht11(DHT11_PIN, DHT11);
String temperature;
String humidity;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true)
;
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(3); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
dht11.begin(); // initialize DHT11 the temperature and humidity sensor
temperature.reserve(10); // to avoid fragmenting memory when using String
humidity.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
float humi = dht11.readHumidity(); // read humidity
float tempC = dht11.readTemperature(); // read temperature
// check if any reads failed
if (isnan(humi) || isnan(tempC)) {
temperature = "Failed";
humidity = "Failed";
} else {
temperature = String(tempC, 1); // one decimal places
temperature += char(247); // degree character
temperature += "C";
humidity = String(humi, 1); // one decimal places
humidity += "%";
}
Serial.print(tempC); // print to Serial Monitor
Serial.print("°C | " ); // print to Serial Monitor
Serial.print(humi); // print to Serial Monitor
Serial.println("%"); // print to Serial Monitor
oledDisplayCenter(temperature, humidity); // display temperature and humidity on OLED
}
void oledDisplayCenter(String temperature, String humidity) {
int16_t x1;
int16_t y1;
uint16_t width_T;
uint16_t height_T;
uint16_t width_H;
uint16_t height_H;
oled.getTextBounds(temperature, 0, 0, &x1, &y1, &width_T, &height_T);
oled.getTextBounds(temperature, 0, 0, &x1, &y1, &width_H, &height_H);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width_T) / 2, SCREEN_HEIGHT / 2 - height_T - 5);
oled.println(temperature); // text to display
oled.setCursor((SCREEN_WIDTH - width_H) / 2, SCREEN_HEIGHT / 2 + 5);
oled.println(humidity); // text to display
oled.display();
}
자세한 사용 방법
다음 지침을 단계별로 따르세요:
- Arduino Uno R4 WiFi/Minima를 처음 사용하는 경우, 아두이노 우노 R4 - 소프트웨어 설치을 참조하세요.
- 제공된 다이어그램에 따라 Arduino Uno R4 보드를 DHT11 온습도 센서 모듈 및 OLED 디스플레이에 연결하세요.
- USB 케이블을 사용하여 Arduino Uno R4 보드를 컴퓨터에 연결하세요.
- 컴퓨터에서 Arduino IDE를 실행하세요.
- 적절한 Arduino Uno R4 보드(예: Arduino Uno R4 WiFi)와 COM 포트를 선택하세요.
- Arduino IDE의 왼쪽에서 Libraries 아이콘으로 이동하세요.
- 검색 상자에 "SSD1306"을 입력한 다음, Adafruit의 SSD1306 라이브러리를 찾으세요.
- Install 버튼을 눌러 라이브러리를 추가하세요.
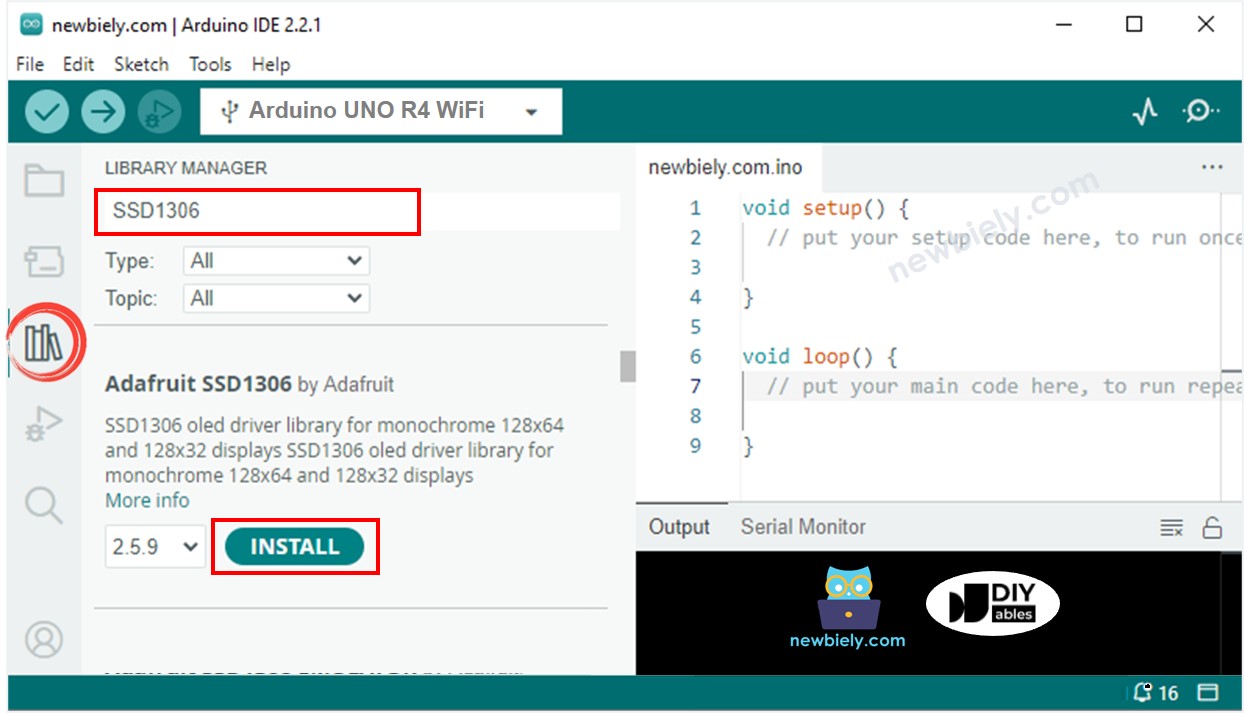
- 추가 라이브러리 종속성을 설치해야 합니다.
- 모든 라이브러리 종속성을 설치하려면 Install All 버튼을 클릭하세요.
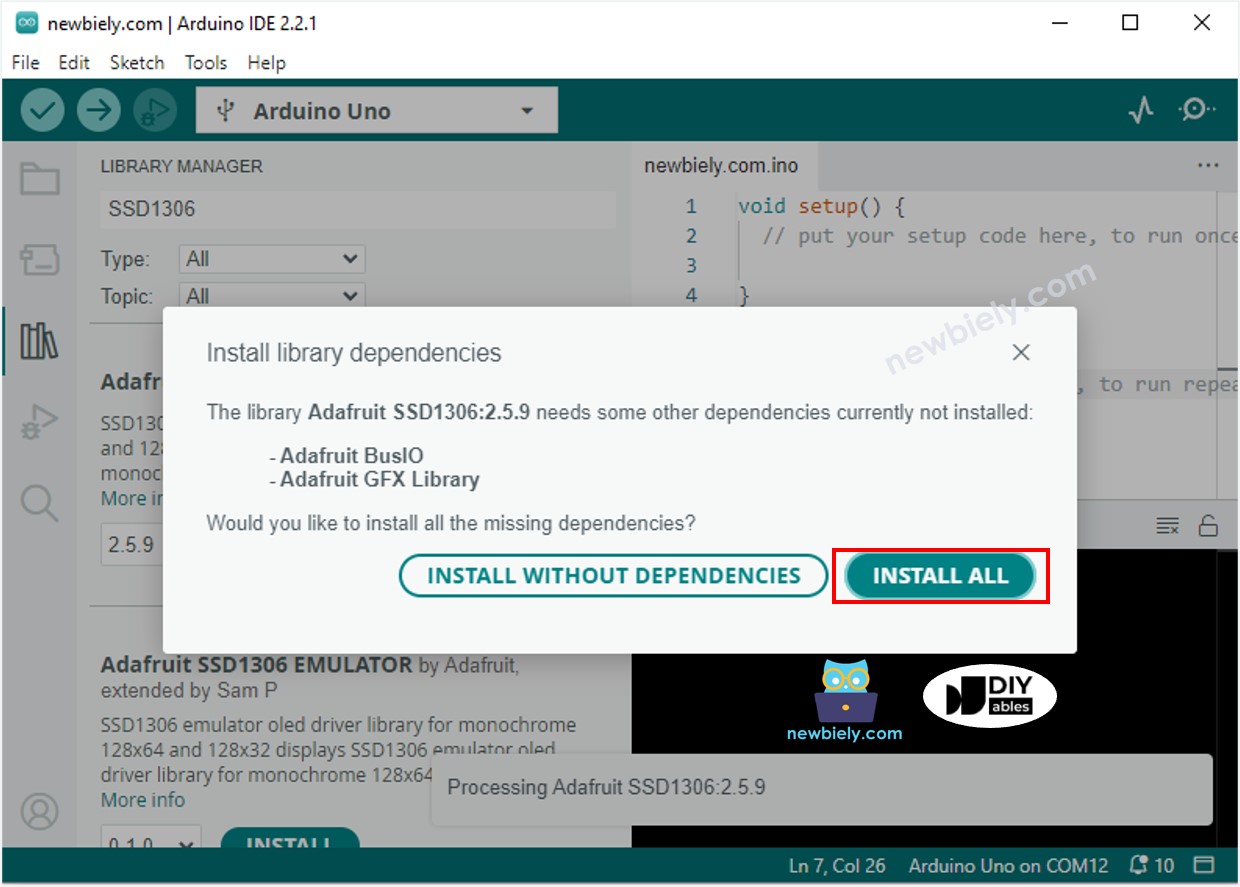
- "DHT"를 검색한 다음, Adafruit에서 만든 DHT 센서 라이브러리를 찾으세요.
- 라이브러리를 추가하려면 Install 버튼을 누르세요.
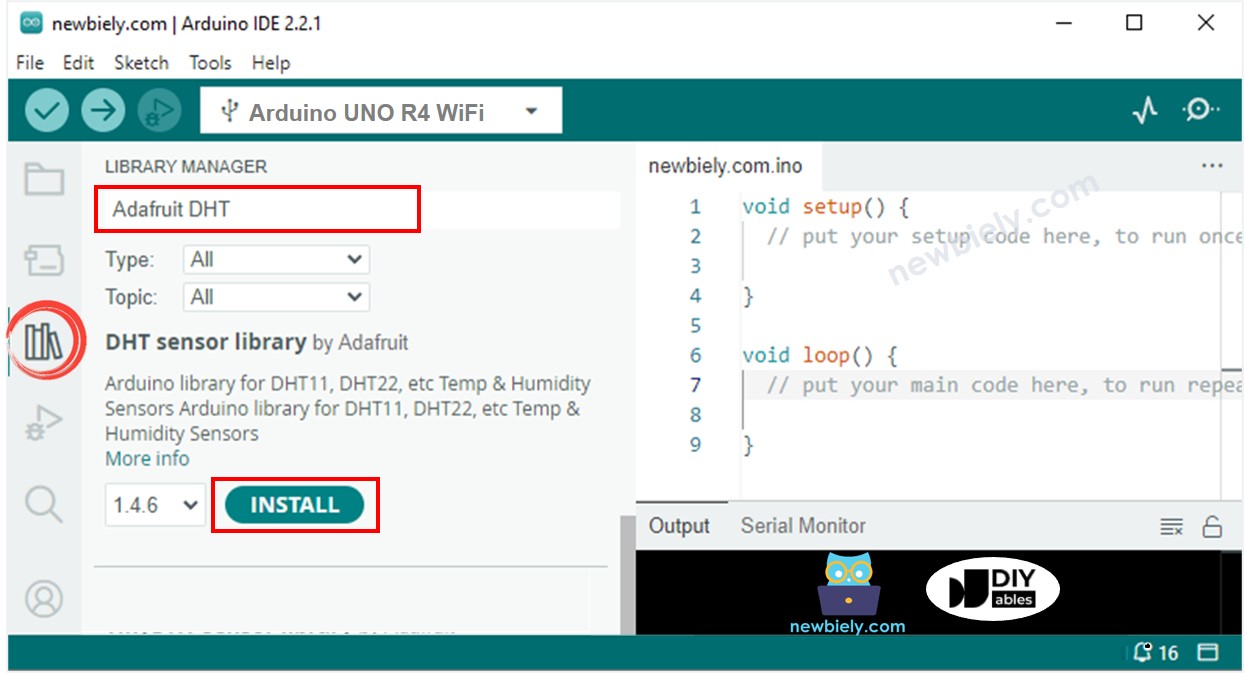
- 추가 라이브러리 종속성을 설치해야 합니다.
- 모든 라이브러리 종속성을 설치하려면 Install All 버튼을 클릭하세요.
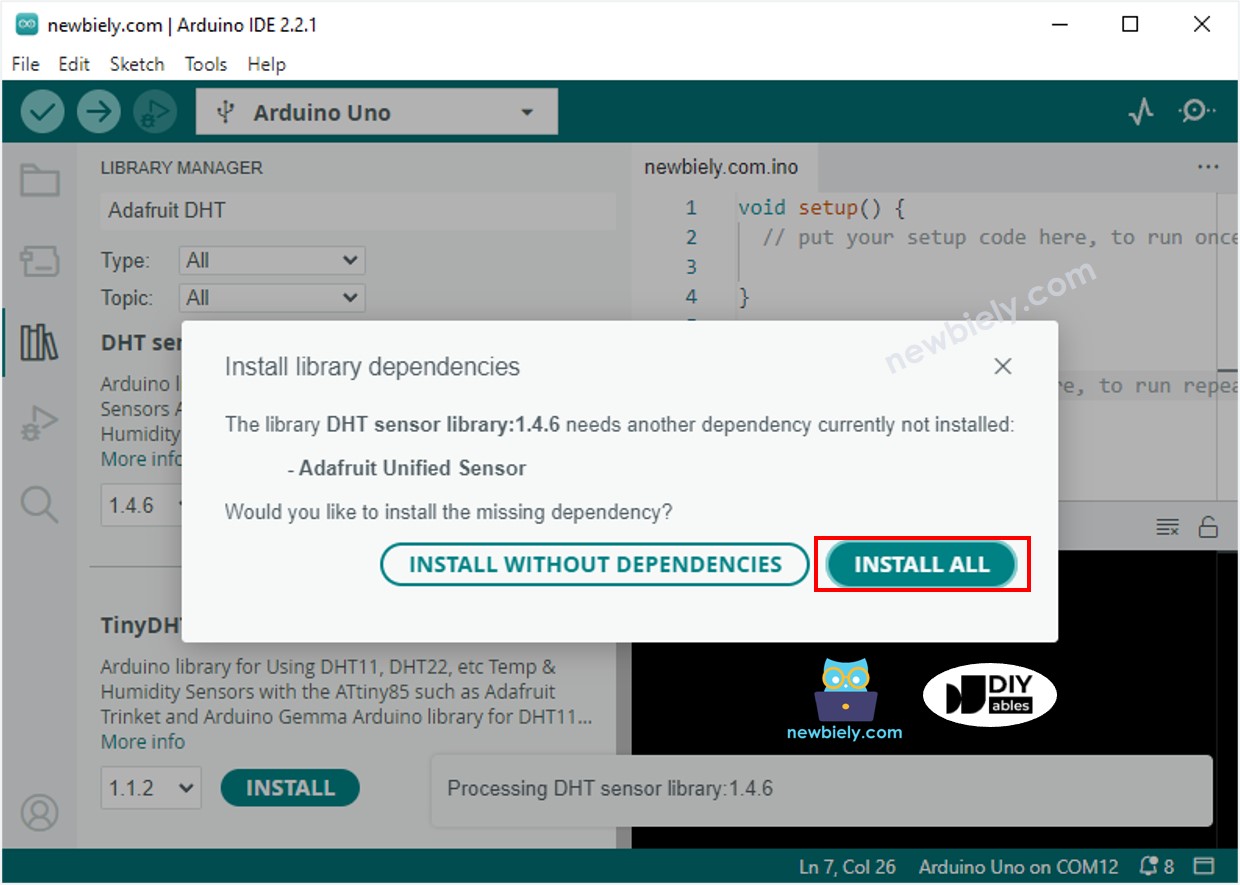
- 위의 코드를 복사하여 Arduino IDE에서 엽니다
- Arduino 툴팁 상단 가이드에서 Upload 버튼을 클릭하여 코드를 Arduino UNO R4에 업로드합니다
- 센서를 뜨거운 물이나 찬물에 넣거나 손에 잡고 있습니다
- OLED 디스플레이와 직렬 모니터에서 결과를 확인합니다
※ 주의:
코드는 OLED 디스플레이에 텍스트를 자동으로 수평 및 수직 중앙에 배치합니다.
동영상
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.