ESP32 코드 구조
ESP32 프로그래밍을 배우려면 ESP32 코드의 구조를 배워야 합니다. 이 튜토리얼은 ESP32 코드의 구조를 제공하고 설명합니다.
준비물
1 | × | ESP32 ESP-WROOM-32 개발 모듈 | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-C | 쿠팡 | 아마존 | |
1 | × | (옵션) DC 커넥터 전원 연결 잭 플러그 소켓 | 쿠팡 | 아마존 | |
1 | × | 브레드보드 | 쿠팡 | 아마존 | |
1 | × | 점퍼케이블 | 아마존 | |
1 | × | (추천) ESP32용 스크루 터미널 확장 보드 | 쿠팡 | 아마존 | |
1 | × | (추천) ESP32용 전원 분배기 | 쿠팡 | 아마존 |
공개: 이 섹션에서 제공된 링크 중 일부는 제휴 링크입니다. 이 링크를 통해 구매한 경우 추가 비용없이 수수료를 받을 수 있습니다. 지원해 주셔서 감사합니다.
기본 코드 구조
ESP32 코드(ESP32 스케치라고도 함)는 아두이노 코드와 동일한 구조를 가집니다. 이것은 두 가지 주요 부분을 포함합니다: 설정 코드와 루프 코드.
설치 코드
- setup() 함수 안에 있는 코드를 설정 코드라고 합니다.
- 설정 코드는 전원을 켜거나 리셋 직후에 실행됩니다.
- 설정 코드는 오직 한 번만 실행됩니다.
- 설정 코드는 변수를 초기화하거나, 핀 모드를 설정하거나, 라이브러리 사용을 시작하는 데에 사용됩니다.
루프 코드
- 루프 코드는 loop() 함수 안에 있는 코드입니다.
- 루프 코드는 설정 코드 바로 뒤에 실행됩니다.
- 루프 코드는 반복적으로 (무한히) 실행됩니다.
- 루프 코드는 애플리케이션의 주된 작업을 수행하는 데 사용됩니다.
예시
void setup() {
// 설정 코드 여기에 작성, 한 번만 실행됩니다:
Serial.begin(9600);
Serial.println("This is ESP32 setup code");
}
void loop() {
// 메인 코드를 여기에 작성, 반복적으로 실행됩니다:
Serial.println("This is ESP32 loop code");
delay(1000);
}
사용 방법
- ESP32를 처음 사용하는 경우, ESP32 - 소프트웨어 설치을 참고하세요.
- 위의 코드를 복사하여 Arduino IDE에 붙여넣으세요.
- Arduino IDE에서 Upload 버튼을 클릭하여 ESP32 보드에 코드를 컴파일하고 업로드하세요.
- Arduino IDE에서 시리얼 모니터를 엽니다.
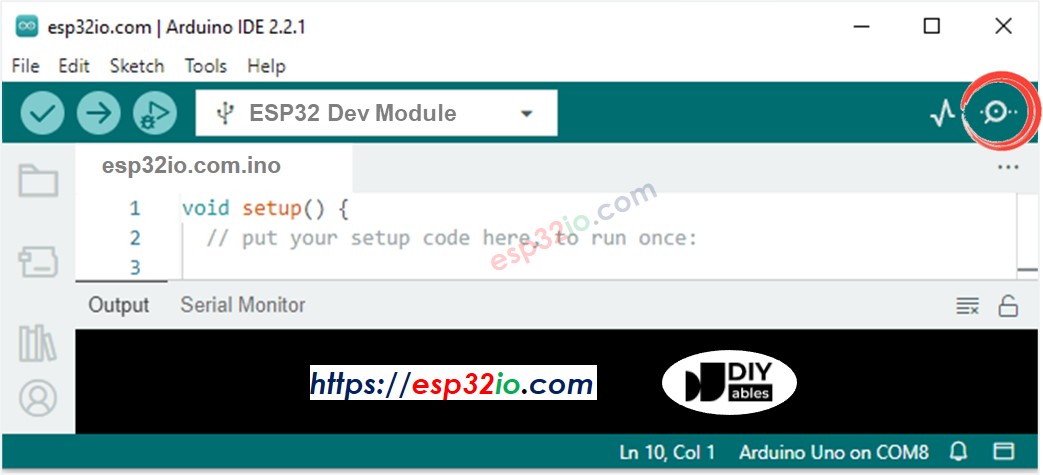
시리얼 모니터에서 출력을 확인하세요.
COM6
This is ESP32 setup code
This is ESP32 loop code
This is ESP32 loop code
This is ESP32 loop code
This is ESP32 loop code
This is ESP32 loop code
This is ESP32 loop code
This is ESP32 loop code
Autoscroll
Clear output
9600 baud
Newline
시리얼 모니터에서 볼 수 있듯이, “이것은 ESP32의 설정 코드입니다”는 한 번 출력되지만, “이것은 ESP32 루프 코드입니다”는 여러 번 출력됩니다. 이는 ESP32 설정 코드가 한 번 실행되고, ESP32 루프 코드는 반복적으로 실행된다는 것을 의미합니다. 설정 코드가 먼저 실행됩니다.
※ 주의:
setup() 및 loop() 함수는 ESP32 코드에서 반드시 사용되어야 합니다. 그렇지 않으면 오류가 발생합니다.
기타 부품
설정 및 루프 코드 외에, ESP32 스케치는 다음과 같은 몇 가지 부분을 포함할 수 있습니다:
- Block comment: usually used to write some information about the author, the wiring instruction, the license ... ESP32 will ignore this part.
- Libraries inclusion: is used to include libraries into the sketch.
- Constant definition: used to define constant
- Global variables declaration
예를 들면:
/*
* 이 ESP32 코드는 newbiely.kr 에서 개발되었습니다
* 이 ESP32 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/esp32/esp32-code-structure
*/
#include <Servo.h>
#include <LiquidCrystal.h>
#define MAX_COUNT 180
Servo servo;
LiquidCrystal lcd(3, 4, 5, 6, 7, 8);
int loop_count = 0;
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
servo.attach(9);
Serial.println("This is ESP32 setup code"); // "이것은 ESP32 설정 코드입니다"
}
void loop() {
loop_count++;
Serial.print("This is ESP32 loop code, count: "); // "이것은 ESP32 루프 코드, 카운트: "
Serial.println(loop_count);
lcd.print("Hello World!");
servo.write(loop_count);
if(loop_count >= MAX_COUNT)
loop_count = 0;
delay(1000);
}
사용 방법
- ESP32를 처음 사용하는 경우, ESP32 - 소프트웨어 설치을 확인하세요.
- 위의 코드를 복사하여 Arduino IDE에 붙여넣으세요.
- Arduino IDE에서 Upload 버튼을 클릭하여 ESP32 보드에 코드를 컴파일하고 업로드하세요.
- Arduino IDE에서 시리얼 모니터를 엽니다.
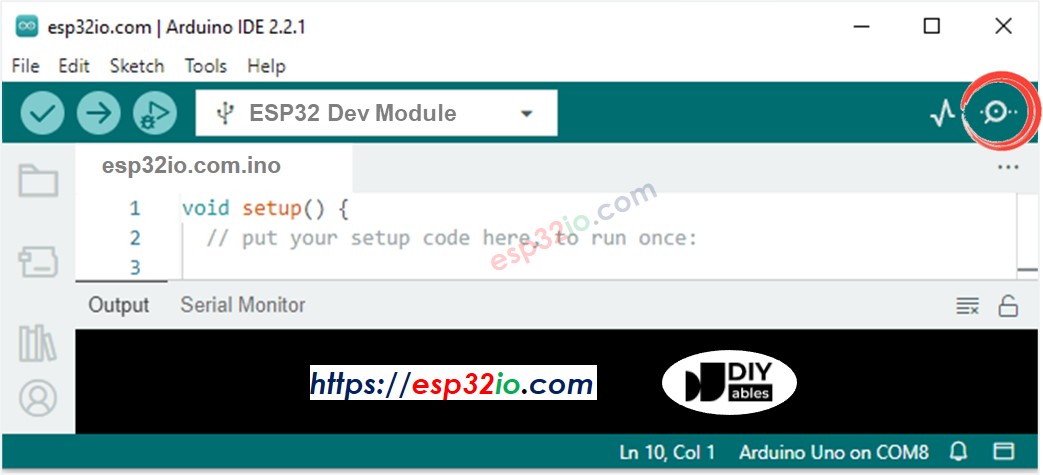
시리얼 모니터에서 출력을 확인하세요.
COM6
This is ESP32 setup code
This is ESP32 loop code, count: 1
This is ESP32 loop code, count: 2
This is ESP32 loop code, count: 3
This is ESP32 loop code, count: 4
This is ESP32 loop code, count: 5
This is ESP32 loop code, count: 6
This is ESP32 loop code, count: 7
Autoscroll
Clear output
9600 baud
Newline
우리는 지금 코드를 줄마다 이해할 필요가 없습니다. 우리는 단지 코드 구조에 대해 알아야 합니다. 줄별 코드는 다음 튜토리얼에서 설명될 것입니다.