ESP32 웹을 통한 온도
이 가이드에서는 ESP32를 웹 서버로 기능하도록 프로그래밍하는 과정을 탐색할 것입니다. 이를 통해 웹 인터페이스를 통해 온도 데이터에 접근할 수 있습니다. 부착된 DS18B20 온도 센서를 사용하여 스마트폰이나 PC로 ESP32가 제공하는 웹 페이지를 방문함으로써 현재 온도를 쉽게 확인할 수 있습니다. 작동 방식에 대한 간략한 개요는 다음과 같습니다:
우리는 두 가지 예제 코드를 살펴볼 것입니다:
DS18B20 센서로부터의 온도를 보여주는 아주 간단한 웹 페이지를 제공하는 ESP32 코드입니다. 이를 통해 작동 방식을 쉽게 이해할 수 있습니다. HTML 내용은 ESP32 코드에 내장되어 있습니다.
DS18B20 센서로부터의 온도를 보여주는 그래픽 웹 페이지를 제공하는 ESP32 코드로, HTML 내용이 ESP32 코드와 분리되어 있습니다.
1 | × | ESP32 ESP-WROOM-32 개발 모듈 | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-A to 타입-C (USB-A PC용) | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-C to 타입-C (USB-C PC용) | 아마존 | |
1 | × | DS18B20 온도 센서 (어댑터 포함) | 쿠팡 | 아마존 | |
1 | × | DS18B20 온도 센서(어댑터 없음) | 아마존 | |
1 | × | 점퍼케이블 | 쿠팡 | 아마존 | |
1 | × | (추천) ESP32용 스크루 터미널 확장 보드 | 쿠팡 | 아마존 | |
1 | × | (추천) ESP32용 전원 분배기 | 쿠팡 | 아마존 | |
공개: 이 포스팅 에 제공된 일부 링크는 아마존 제휴 링크입니다. 이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다.
ESP32 웹 서버와 DS18B20 온도 센서(핀 배치, 작동 방식, 프로그래밍 방법 등)에 대해 잘 모른다면, 다음 튜토리얼에서 배워보세요:
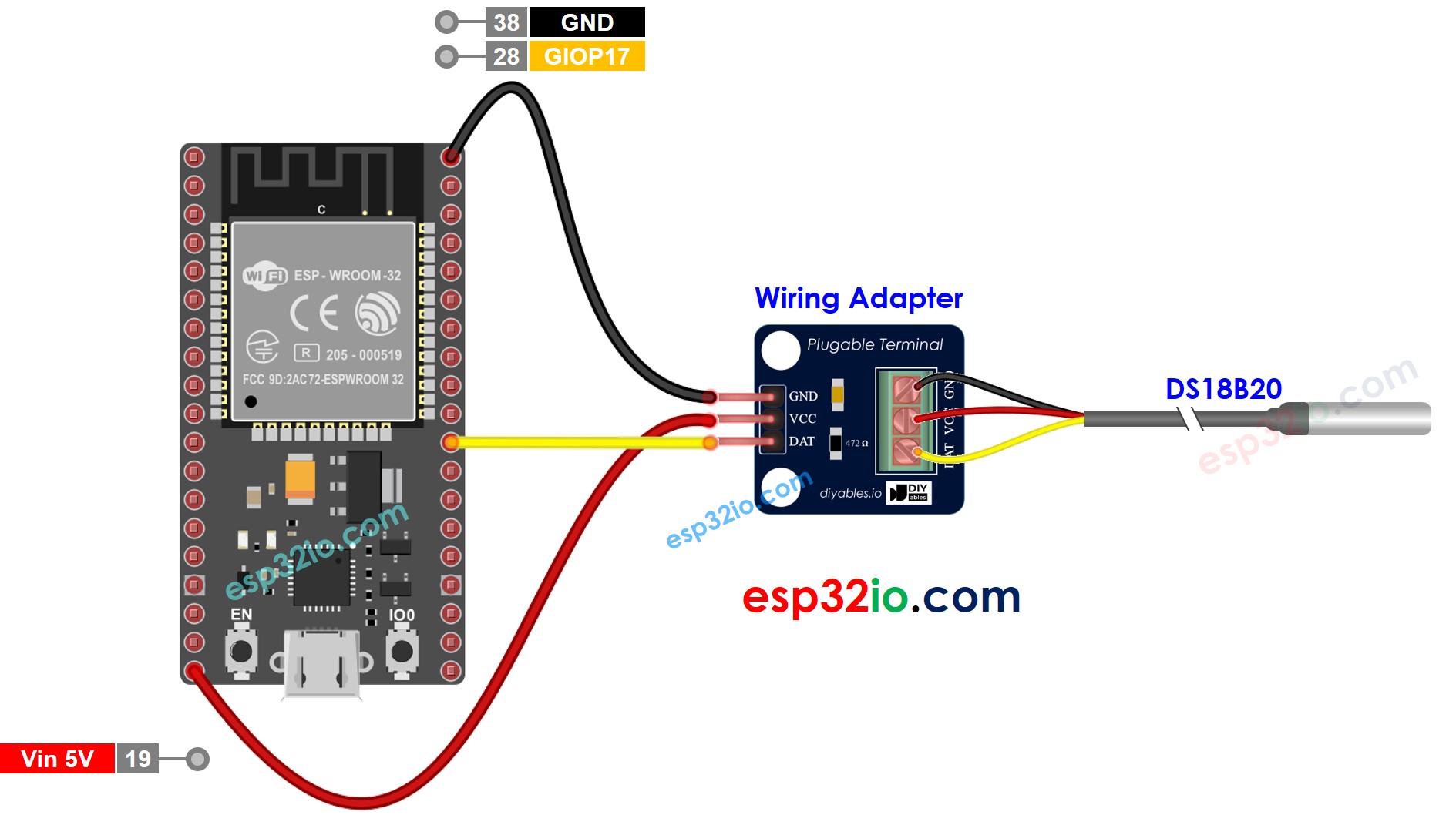
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
ESP32 및 다른 구성 요소에 전원을 공급하는 방법에 대해 잘 알지 못하는 경우, 다음 튜토리얼에서 안내를 찾을 수 있습니다: ESP32 전원 공급 방법.
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#define SENSOR_PIN 17
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
OneWire oneWire(SENSOR_PIN);
DallasTemperature DS18B20(&oneWire);
AsyncWebServer server(80);
float getTemperature() {
DS18B20.requestTemperatures();
float tempC = DS18B20.getTempCByIndex(0);
return tempC;
}
void setup() {
Serial.begin(9600);
DS18B20.begin();
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("ESP32 Web Server's IP address: ");
Serial.println(WiFi.localIP());
server.on("/", HTTP_GET, [](AsyncWebServerRequest* request) {
Serial.println("ESP32 웹 서버: 새 요청 수신됨:");
Serial.println("GET /");
float temperature = getTemperature();
String temperatureStr = String(temperature, 2);
String html = "<!DOCTYPE HTML>";
html += "<html>";
html += "<head>";
html += "<link rel=\"icon\" href=\"data:,\">";
html += "</head>";
html += "<p>";
html += "Temperature: <span style=\"color: red;\">";
html += temperature;
html += "°C</span>";
html += "</p>";
html += "</html>";
request->send(200, "text/html", html);
});
server.begin();
}
void loop() {
}
위 이미지와 같이 배선하세요.
ESP32 보드를 마이크로 USB 케이블을 이용해 PC에 연결하세요.
PC에서 Arduino IDE를 엽니다.
올바른 ESP32 보드(예: ESP32 Dev Module)와 COM 포트를 선택하세요.
Arduino IDE의 왼쪽 네비게이션 바에서 Library Manager 아이콘을 클릭해 라이브러리 관리자를 엽니다.
“ESPAsyncWebServer”를 검색하고, lacamera가 만든 ESPAsyncWebServer를 찾으세요.
Install 버튼을 클릭해 ESPAsyncWebServer 라이브러리를 설치하세요.
종속성을 설치하라는 요청을 받게 됩니다. Install All 버튼을 클릭하세요.
검색 창에서 “DallasTemperature”을 검색한 다음, Miles Burton의 DallasTemperature 라이브러리를 찾아보세요.
DallasTemperature 라이브러리를 설치하려면 Install 버튼을 클릭하세요.
의존성을 설치하라는 요청을 받게 됩니다. OneWire 라이브러리를 설치하려면 Install All 버튼을 클릭하세요.
Connecting to WiFi...
Connected to WiFi
ESP32 Web Server's IP address: 192.168.0.2
Connecting to WiFi...
Connected to WiFi
ESP32 Web Server's IP address: 192.168.0.2
ESP32 Web Server: New request received:
GET /
아래와 같이 웹 브라우저에서 ESP32 보드의 매우 간단한 웹 페이지를 볼 수 있습니다:
※ 주의:
위에 제공된 코드를 사용하여 온도 업데이트를 받으려면 웹 브라우저에서 페이지를 다시 로드해야 합니다. 다음 부분에서는 웹 페이지를 다시 로드하지 않고 배경에서 온도 값을 업데이트하는 방법을 배우게 됩니다.
그래픽 웹 페이지에는 많은 양의 HTML 콘텐츠가 포함되어 있으므로 이전과 같이 ESP32 코드에 내장하는 것이 불편해집니다. 이 문제를 해결하기 위해, ESP32 코드와 HTML 코드를 다른 파일로 분리할 필요가 있습니다:
ESP32 코드는 .ino 파일에 위치할 것입니다.
HTML 코드(HTML, CSS, 및 Javascript를 포함)는 .h 파일에 위치할 것입니다.
HTML 코드를 ESP32 코드에서 분리하는 방법에 대한 자세한 내용은 ESP32 - 웹 서버 튜토리얼을 참조하십시오.
#include <WiFi.h>
#include <ESPAsyncWebServer.h>
#include "index.h"
#include <OneWire.h>
#include <DallasTemperature.h>
#define SENSOR_PIN 17
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
OneWire oneWire(SENSOR_PIN);
DallasTemperature DS18B20(&oneWire);
AsyncWebServer server(80);
float getTemperature() {
DS18B20.requestTemperatures();
float tempC = DS18B20.getTempCByIndex(0);
return tempC;
}
void setup() {
Serial.begin(9600);
DS18B20.begin();
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.print("ESP32 Web Server's IP address: ");
Serial.println(WiFi.localIP());
server.on("/", HTTP_GET, [](AsyncWebServerRequest* request) {
Serial.println("ESP32 Web Server: New request received:");
Serial.println("GET /");
request->send(200, "text/html", webpage);
});
server.on("/temperature", HTTP_GET, [](AsyncWebServerRequest* request) {
Serial.println("ESP32 Web Server: New request received:");
Serial.println("GET /temperature");
float temperature = getTemperature();
String temperatureStr = String(temperature, 2);
request->send(200, "text/plain", temperatureStr);
});
server.begin();
}
void loop() {
}
코드에서 WiFi 정보 (SSID 및 비밀번호)를 귀하의 것으로 변경하세요.
아두이노 IDE에서 index.h 파일을 생성하는 방법:
파일 이름을 index.h로 지정하고 OK 버튼을 클릭하세요.
아래 코드를 복사해서 index.h에 붙여넣으세요.
#ifndef WEBPAGE_H
#define WEBPAGE_H
const char* webpage = R"=====(
<!DOCTYPE html>
<html>
<head>
<title>ESP32 - Web Temperature</title>
<meta name="viewport" content="width=device-width, initial-scale=0.7, maximum-scale=0.7">
<meta charset="utf-8">
<link rel="icon" href="https://diyables.io/images/page/diyables.svg">
<style>
body { font-family: "Georgia"; text-align: center; font-size: width/2pt;}
h1 { font-weight: bold; font-size: width/2pt;}
h2 { font-weight: bold; font-size: width/2pt;}
button { font-weight: bold; font-size: width/2pt;}
</style>
<script>
var cvs_width = 200, cvs_height = 450;
function init() {
var canvas = document.getElementById("cvs");
canvas.width = cvs_width;
canvas.height = cvs_height + 50;
var ctx = canvas.getContext("2d");
ctx.translate(cvs_width/2, cvs_height - 80);
fetchTemperature();
setInterval(fetchTemperature, 4000);
}
function fetchTemperature() {
fetch("/temperature")
.then(response => response.text())
.then(data => {update_view(data);});
}
function update_view(temp) {
var canvas = document.getElementById("cvs");
var ctx = canvas.getContext("2d");
var radius = 70;
var offset = 5;
var width = 45;
var height = 330;
ctx.clearRect(-cvs_width/2, -cvs_height + 80, cvs_width, cvs_height + 50);
ctx.strokeStyle="blue";
ctx.fillStyle="blue";
var x = -width/2;
ctx.lineWidth=2;
for (var i = 0; i <= 100; i+=5) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 20, y);
ctx.stroke();
}
ctx.lineWidth=5;
for (var i = 0; i <= 100; i+=20) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 25, y);
ctx.stroke();
ctx.font="20px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="right";
ctx.fillText(i.toString(), x - 35, y);
}
ctx.lineWidth=16;
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.stroke();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.stroke();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.stroke();
ctx.fillStyle="#e6e6ff";
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.fill();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.fill();
ctx.fillStyle="#ff1a1a";
ctx.beginPath();
ctx.arc(0, 0, radius - offset, 0, 2 * Math.PI);
ctx.fill();
temp = Math.round(temp * 100) / 100;
var y = (height - radius)*temp/100.0 + radius + 5;
ctx.beginPath();
ctx.rect(-width/2 + offset, -y, width - 2*offset, y);
ctx.fill();
ctx.fillStyle="red";
ctx.font="bold 34px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="center";
ctx.fillText(temp.toString() + "°C", 0, 100);
}
window.onload = init;
</script>
</head>
<body>
<h1>ESP32 - Web Temperature</h1>
<canvas id="cvs"></canvas>
</body>
</html>
)=====";
#endif
이제 두 개의 파일에 코드를 가지고 있습니다: newbiely.kr.ino 및 index.h
Arduino IDE에서 Upload 버튼을 클릭하여 ESP32에 코드를 업로드하세요.
이전과 같이 PC나 스마트폰의 웹 브라우저를 통해 ESP32 보드의 웹 페이지에 접속하세요. 아래와 같이 보일 것입니다:
※ 주의:
index.h의 HTML 내용을 수정하고 newbiely.kr.ino 파일을 건드리지 않는 경우, 코드를 ESP32에 컴파일하고 업로드할 때 Arduino IDE는 HTML 내용을 업데이트하지 않습니다.
이 경우 Arduino IDE가 HTML 내용을 업데이트하게 하려면, newbiely.kr.ino 파일을 변경해야 합니다 (예: 빈 줄 추가, 주석 추가...).