ESP32 이더넷
이 튜티리얼은 W5500 이더넷 모듈을 사용하여 ESP32를 인터넷 또는 LAN 네트워크에 연결하는 방범을 안내합니다. 자세히 배우게 될 내용은 다음과 같습니다:
- ESP32를 W5500 이더넷 모듈에 연결하는 방벅
- 이더넷을 통해 HTTP 요청을 하기 위해 ESP32를 프로그램하는 방법
- 이더넷을 통해 간단한 웹 서버를 생성하기 위해 ESP32를 프로그램하는 방법
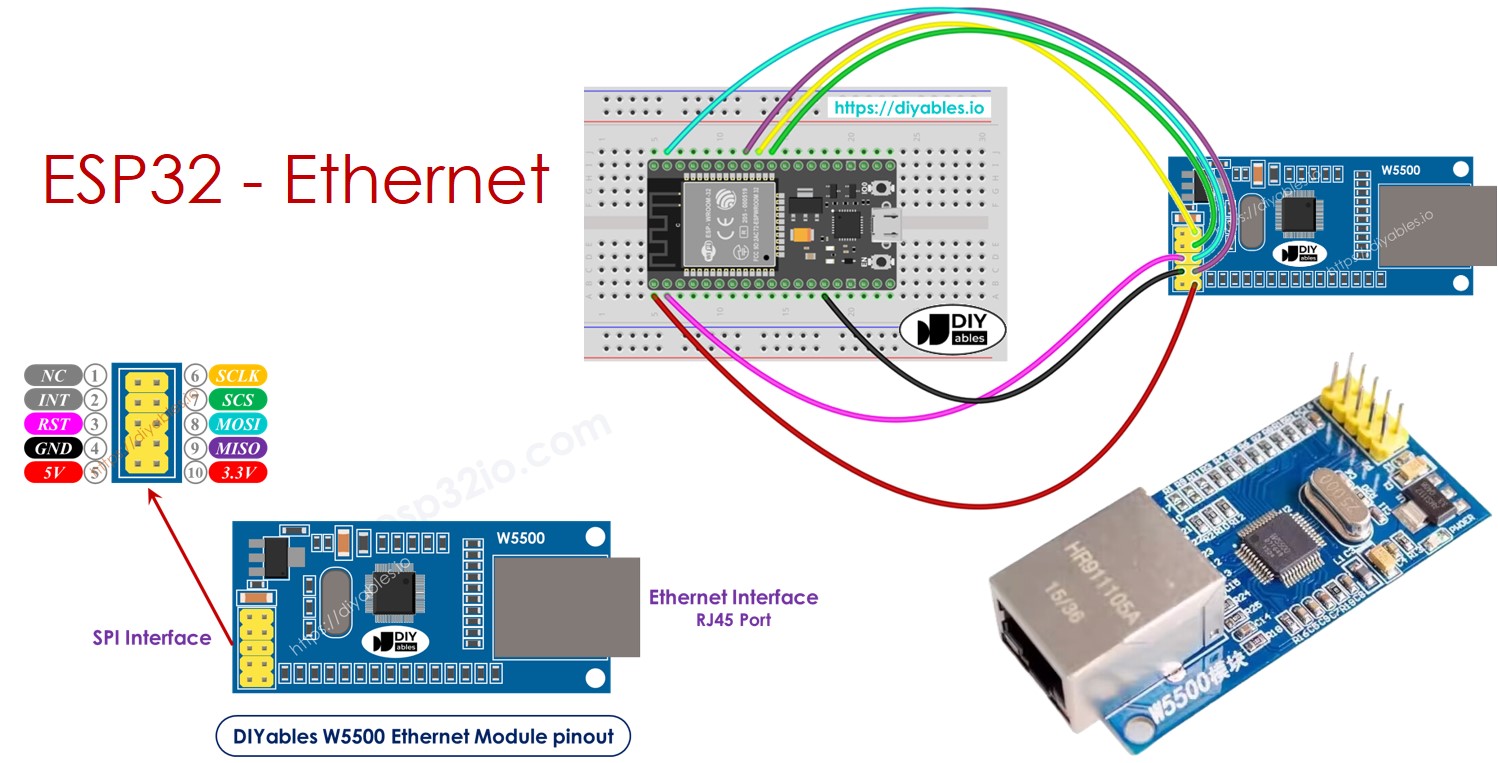
준비물
1 | × | ESP32 ESP-WROOM-32 개발 모듈 | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-A to 타입-C (USB-A PC용) | 쿠팡 | 아마존 | |
1 | × | USB 케이블 타입-C to 타입-C (USB-C PC용) | 아마존 | |
1 | × | W5500 Ethernet Module | 쿠팡 | 아마존 | |
1 | × | Ethernet Cable | 쿠팡 | 아마존 | |
1 | × | 점퍼케이블 | 쿠팡 | 아마존 | |
1 | × | 브레드보드 | 쿠팡 | 아마존 | |
1 | × | (추천) ESP32용 스크루 터미널 확장 보드 | 쿠팡 | 아마존 | |
1 | × | (추천) ESP32용 전원 분배기 | 쿠팡 | 아마존 |
공개: 이 포스팅 에 제공된 일부 링크는 아마존 제휴 링크입니다. 이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다.
W5500 이더넷 모듈에 대하여
W5500 이더넷 모듈에는 두 가지 인터페이스가 있습니다.
- RJ45 인터페이스: 이더넷 케이블을 통해 라우터/스위치에 연결합니다.
- SPI 인터페이스: ESP32 보드에 연결하며, 10개의 핀을 포함합니다:
- NC 핀: 이 핀은 연결하지 않습니다.
- INT 핀: 이 핀은 연결하지 않습니다.
- RST 핀: 리셋 핀, 이 핀을 ESP32의 EN 핀에 연결합니다.
- GND 핀: 이 핀을 ESP32의 GND 핀에 연결합니다.
- 5V 핀: 이 핀은 연결하지 않습니다.
- 3.3V 핀: 이 핀을 ESP32의 3.3V 핀에 연결합니다.
- MISO 핀: 이 핀을 ESP32의 SPI MISO 핀에 연결합니다.
- MOSI 핀: 이 핀을 ESP32의 SPI MOSI 핀에 연결합니다.
- SCS 핀: 이 핀을 ESP32의 SPI CS 핀에 연결합니다.
- SCLK 핀: 이 핀을 ESP32의 SPI SCK 핀에 연결합니다.
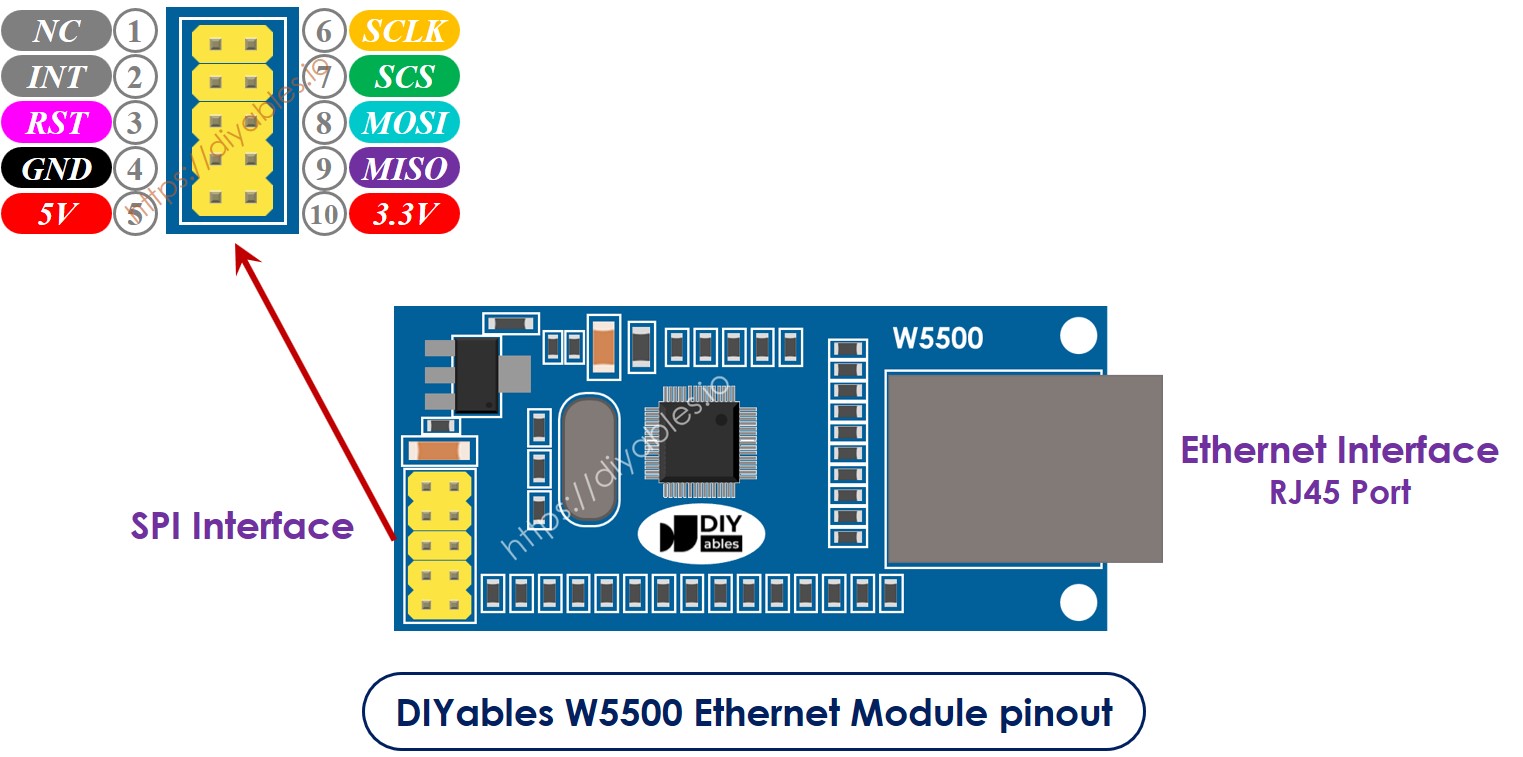
image source: diyables.io
ESP32와 W5500 이더넷 모듈 간의 배선도
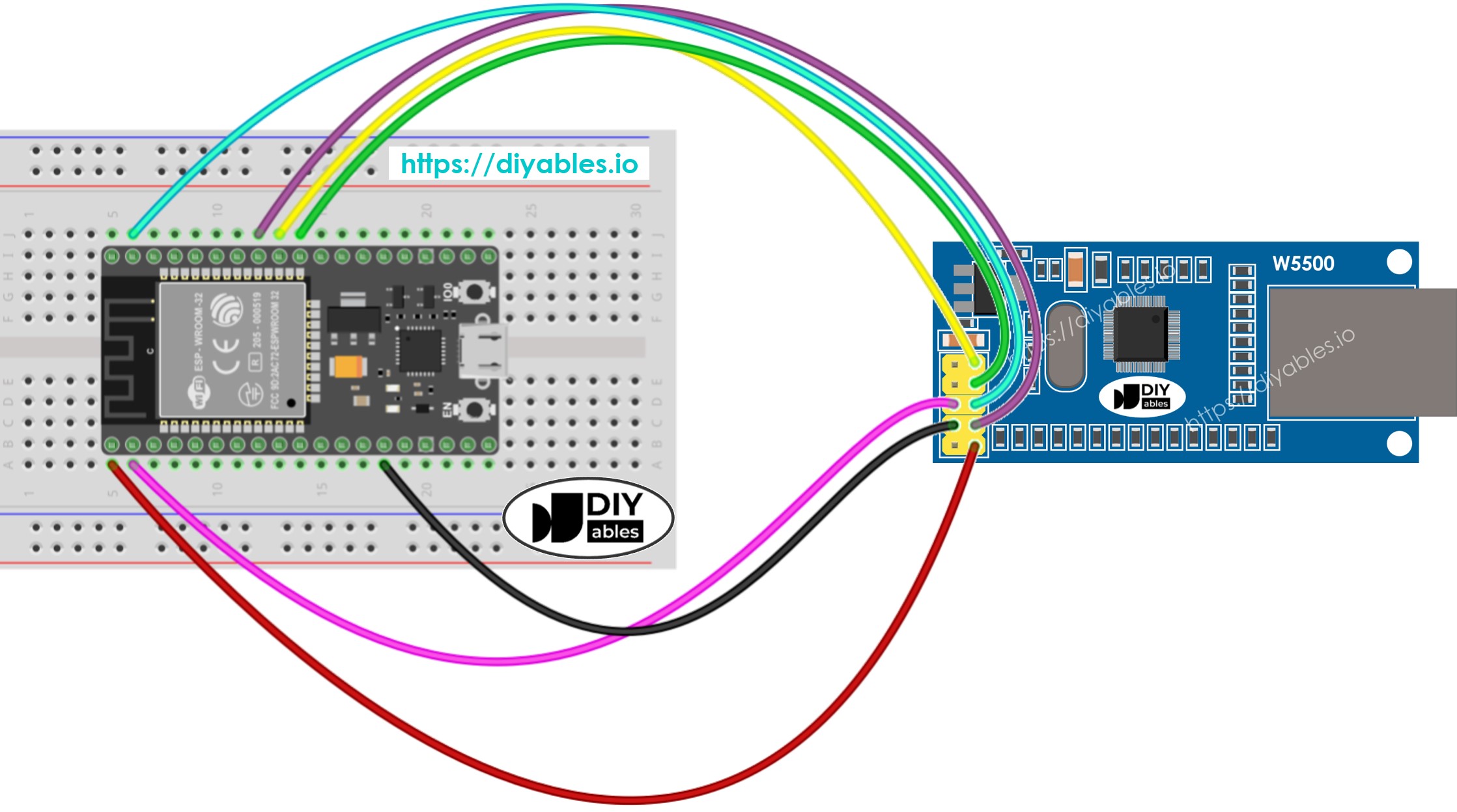
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
ESP32 및 다른 구성 요소에 전원을 공급하는 방법에 대해 잘 알지 못하는 경우, 다음 튜토리얼에서 안내를 찾을 수 있습니다: ESP32 전원 공급 방법.
image source: diyables.io
ESP32 코드 - 이더넷 모듈을 통한 HTTP 요청 실행
다음 코드는 http://example.com/의 웹 서버에 HTTP 요청을 하는 웹 클라이언트로 기능합니다.
/*
* 이 ESP32 코드는 newbiely.kr 에서 개발되었습니다
* 이 ESP32 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/esp32/esp32-ethernet
*/
#include <SPI.h>
#include <Ethernet.h>
// replace the MAC address below by the MAC address printed on a sticker on the Arduino Shield 2
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetClient client;
int HTTP_PORT = 80;
String HTTP_METHOD = "GET"; // or POST
char HOST_NAME[] = "example.com";
String PATH_NAME = "/";
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("ESP32 - Ethernet Tutorial");
// initialize the Ethernet shield using DHCP:
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
// check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
// check for Ethernet cable
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
// connect to web server on port 80:
if (client.connect(HOST_NAME, HTTP_PORT)) {
// if connected:
Serial.println("Connected to server");
// make a HTTP request:
// send HTTP header
client.println(HTTP_METHOD + " " + PATH_NAME + " HTTP/1.1");
client.println("Host: " + String(HOST_NAME));
client.println("Connection: close");
client.println(); // end HTTP header
while (client.connected()) {
if (client.available()) {
// read an incoming byte from the server and print it to serial monitor:
char c = client.read();
Serial.print(c);
}
}
// the server's disconnected, stop the client:
client.stop();
Serial.println();
Serial.println("disconnected");
} else { // if not connected:
Serial.println("connection failed");
}
}
void loop() {
}
자세한 사용 방법
- ESP32를 처음 사용하는 경우, ESP32 - 소프트웨어 설치을 참조하세요.
- 위의 배선도대로 이더넷 모듈과 ESP32를 연결하세요.
- ESP32를 USB 케이블을 통해 PC에 연결하세요.
- 이더넷 모듈을 이더넷 케이블을 통해 라우터/스위치에 연결하세요.
- PC에서 Arduino IDE를 엽니다.
- 올바른 ESP32 보드(예: ESP32 Dev Module)와 COM 포트를 선택하세요.
- Arduino IDE의 왼쪽 바에 있는 Libraries 아이콘을 클릭하세요.
- “Ethernet”을 검색한 다음, Various가 제공하는 이더넷 라이브러리를 찾으세요.
- Install 버튼을 클릭해서 이더넷 라이브러리를 설치하세요.
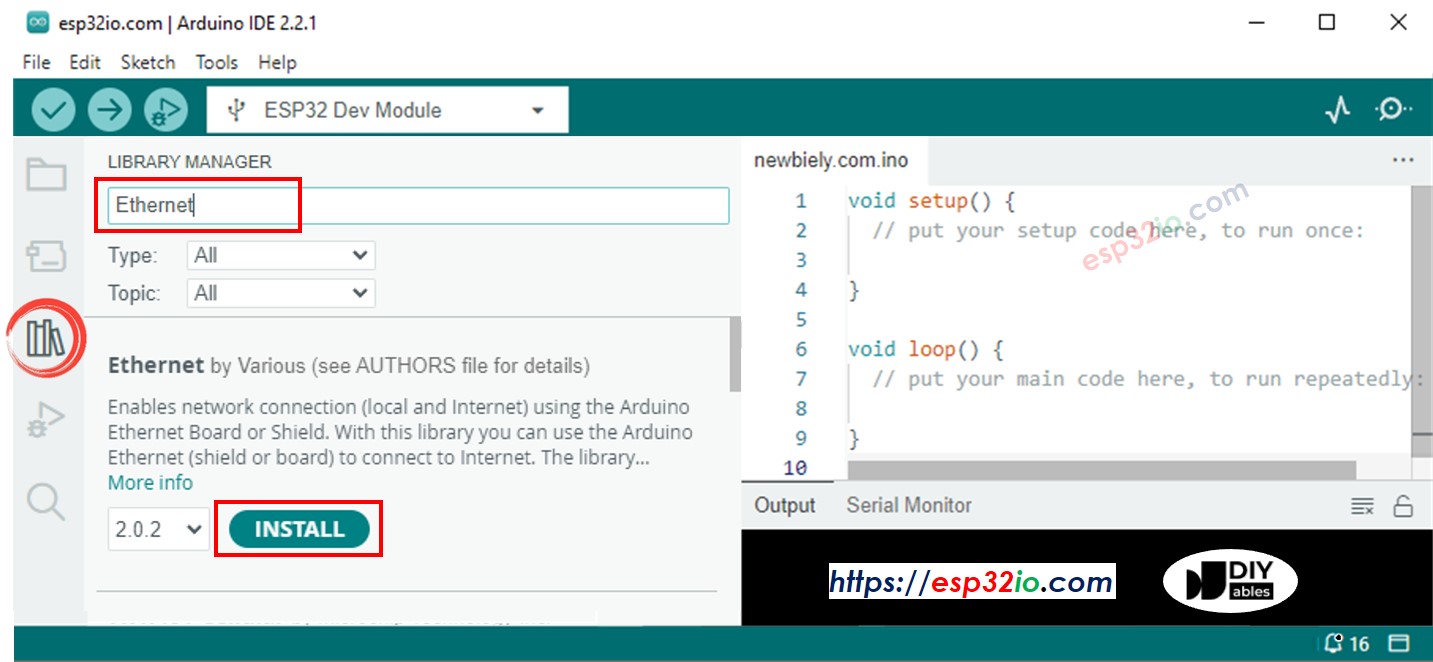
- 아두이노 IDE에서 시리얼 열기
- 위의 코드를 복사하여 아두이노 IDE에 붙여넣기
- 아두이노 IDE에서 Upload 버튼을 클릭하여 코드를 ESP32에 업로드
- 시리얼 모니터에서 결과를 확인하세요. 결과는 다음과 같습니다:
COM6
ESP32 - Ethernet Tutorial
Connected to server
HTTP/1.1 200 OK
Accept-Ranges: bytes
Age: 208425
Cache-Control: max-age=604800
Content-Type: text/html; charset=UTF-8
Date: Fri, 12 Jul 2024 07:08:42 GMT
Etag: "3147526947"
Expires: Fri, 19 Jul 2024 07:08:42 GMT
Last-Modified: Thu, 17 Oct 2019 07:18:26 GMT
Server: ECAcc (lac/55B8)
Vary: Accept-Encoding
X-Cache: HIT
Content-Length: 1256
Connection: close
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
disconnected
Autoscroll
Clear output
9600 baud
Newline
※ 주의:
같은 로컬 네트워크에 동일한 MAC 주소를 가진 다른 기기가 있다면 작동하지 않을 수 있습니다.
ESP32 코드 - 이더넷 모듈용 웹 서버
다음 코드는 ESP32를 웹 브라우저에 간단한 웹페이지로 응답하는 웹 서버로 변환합니다.
/*
* 이 ESP32 코드는 newbiely.kr 에서 개발되었습니다
* 이 ESP32 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/esp32/esp32-ethernet
*/
#include <SPI.h>
#include <Ethernet.h>
// replace the MAC address below by the MAC address printed on a sticker on the Arduino Shield 2
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetServer server(80);
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("ESP32 - Ethernet Tutorial");
// initialize the Ethernet shield using DHCP:
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
// check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
// check for Ethernet cable
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
server.begin();
Serial.print("ESP32 - Web Server IP Address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
// listen for incoming clients
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
// an HTTP request ends with a blank line
bool currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
// if you've gotten to the end of the line (received a newline
// character) and the line is blank, the HTTP request has ended,
// so you can send a reply
if (c == '\n' && currentLineIsBlank) {
// send a standard HTTP response header
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close"); // the connection will be closed after completion of the response
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<body>");
client.println("<h1>ESP32 Web Server with Ethernet</h1>");
client.println("</body>");
client.println("</html>");
break;
}
if (c == '\n') {
// you're starting a new line
currentLineIsBlank = true;
} else if (c != '\r') {
// you've gotten a character on the current line
currentLineIsBlank = false;
}
}
}
// give the web browser time to receive the data
delay(1);
// close the connection:
client.stop();
Serial.println("client disconnected");
}
}
자세한 사용 방법
- 위 코드를 복사하여 아두이노 IDE에 붙여넣으세요
- 아두이노 IDE에서 Upload 버튼을 클릭하여 코드를 ESP32에 업로드하세요
- 시리얼 모니터에서 결과를 확인하세요, 아래와 같이 표시됩니다:
COM6
ESP32 - Ethernet Tutorial
ESP32 - Web Server IP Address: 192.168.0.2
Autoscroll
Clear output
9600 baud
Newline
- 위의 IP 주소를 복사하여 웹 브라우저의 주소창에 붙여넣으면 다음과 같이 ESP32에서 제공하는 간단한 웹페이지를 볼 수 있습니다:
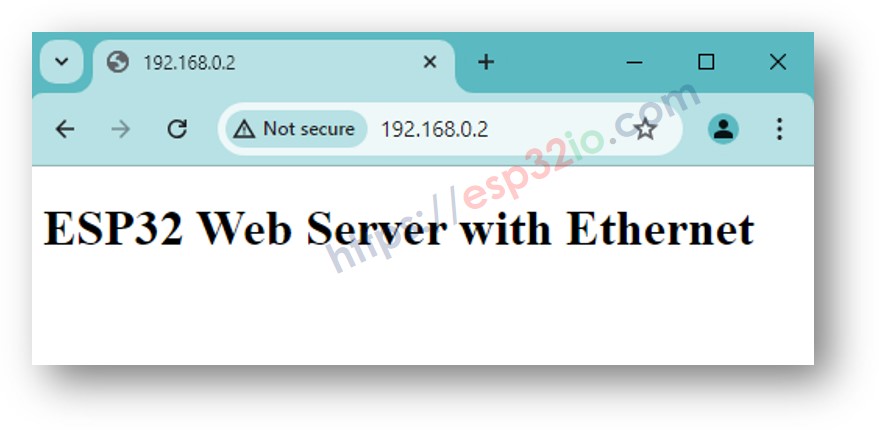