아두이노 여러 버튼
이 튜토리얼은 delay() 함수를 사용하지 않고 여러 버튼을 동시에 사용하도록 Arduino를 프로그래밍하는 방법을 가르쳐줍니다. 이 튜토리얼은 두 가지 방식으로 코드를 제공합니다:
- 아두이노 다중 버튼 디바운싱
- 배열을 사용한 아두이노 다중 버튼 디바운싱.
우리는 예시로 다섯 개의 버튼을 사용할 것입니다. 여러분은 이를 쉽게 변경하여 두 개의 버튼, 네 개의 버튼 또는 그 이상으로 적용할 수 있습니다.
준비물
1 | × | 아두이노 우노 R3 | 쿠팡 | 아마존 | |
1 | × | USB 2.0 타입 A-to-B 케이블 (USB-A PC용) | 쿠팡 | 아마존 | |
1 | × | USB 2.0 타입 C-to-B 케이블 (USB-C PC용) | 아마존 | |
1 | × | 캡이 있는 버튼 | 쿠팡 | 아마존 | |
1 | × | 버튼 키트 | 쿠팡 | 아마존 | |
1 | × | Panel-mount Button | 아마존 | |
1 | × | 푸시 버튼 모듈 | 아마존 | |
1 | × | 브레드보드 | 쿠팡 | 아마존 | |
1 | × | 점퍼케이블 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 스크루 터미널 블록 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 브레드보드 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 케이스 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 프로토타이핑 베이스 플레이트 & 브레드보드 키트 | 아마존 |
공개: 이 포스팅 에 제공된 일부 링크는 아마존 제휴 링크입니다. 이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다.
버튼에 대하여
우리는 하드웨어 핀 배치, 작동 원리, 아두이노 연결 방법, 코드 지침을 포함한 상세한 버튼 튜토리얼을 가지고 있습니다. 자세한 정보는 여기에서 확인하세요:
- 아두이노 - 버튼 튜토리얼
- 아두이노 - 버튼 - 디벗싱 튜토리얼
선연결
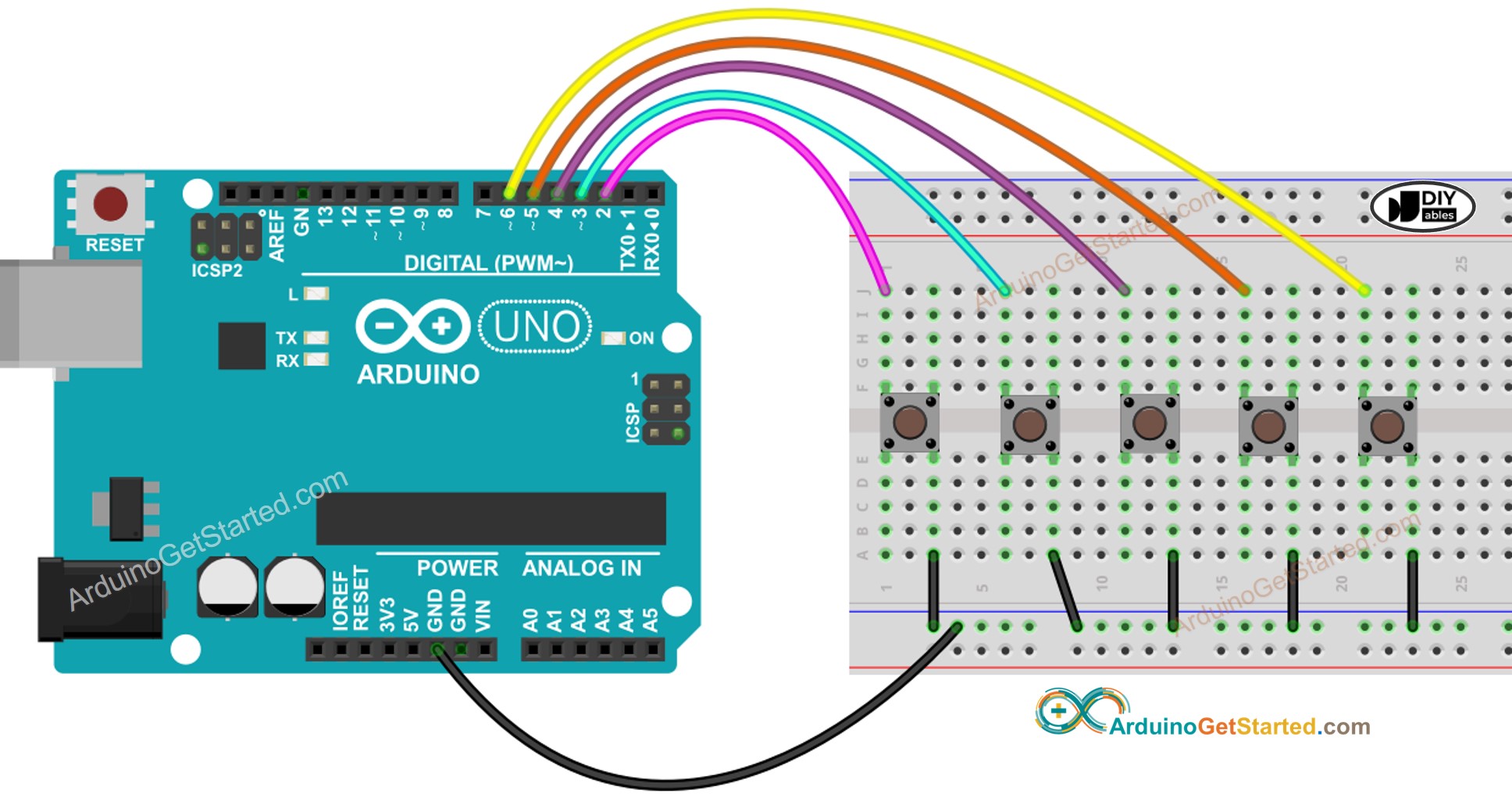
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
아두이노 코드 - 디바운스를 적용한 여러 버튼
여러 버튼을 사용할 때 특정 상황에서 복잡해질 수 있습니다:
- 버튼 디바운싱이 필요한 애플리케이션 (버튼에 디바운싱이 필요한 이유를 보려면 여기를 클릭하세요)
- 상태 변화(눌림/해제)를 감지해야 하는 애플리케이션
다행히도, ezButton 라이브러리는 디바운스와 버튼 이벤트를 내부적으로 관리하여 이 과정을 간소화합니다. 이는 사용자가 라이브러리를 사용할 때 타임스탬프와 변수를 관리하는 작업에서 해방시켜 줍니다. 추가로, 버튼 배열을 사용하는 것은 코드의 명확성과 간결함을 향상시킬 수 있습니다.
/*
* 이 아두이노 코드는 newbiely.kr 에서 개발되었습니다
* 이 아두이노 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-multiple-button
*/
#include <ezButton.h>
#define BUTTON_NUM 5 // 버튼의 수
#define BUTTON_PIN_1 2 // 버튼 1에 연결된 아두이노 핀
#define BUTTON_PIN_2 3 // 버튼 2에 연결된 아두이노 핀
#define BUTTON_PIN_3 4 // 버튼 3에 연결된 아두이노 핀
#define BUTTON_PIN_4 5 // 버튼 4에 연결된 아두이노 핀
#define BUTTON_PIN_5 6 // 버튼 5에 연결된 아두이노 핀
ezButton button1(BUTTON_PIN_1); // 버튼 1을 위한 ezButton 객체 생성
ezButton button2(BUTTON_PIN_2); // 버튼 2를 위한 ezButton 객체 생성
ezButton button3(BUTTON_PIN_3); // 버튼 3을 위한 ezButton 객체 생성
ezButton button4(BUTTON_PIN_4); // 버튼 4를 위한 ezButton 객체 생성
ezButton button5(BUTTON_PIN_5); // 버튼 5를 위한 ezButton 객체 생성
void setup() {
Serial.begin(9600);
button1.setDebounceTime(100); // 디바운스 시간을 100 밀리초로 설정
button2.setDebounceTime(100); // 디바운스 시간을 100 밀리초로 설정
button3.setDebounceTime(100); // 디바운스 시간을 100 밀리초로 설정
button4.setDebounceTime(100); // 디바운스 시간을 100 밀리초로 설정
button5.setDebounceTime(100); // 디바운스 시간을 100 밀리초로 설정
}
void loop() {
button1.loop(); // loop() 함수를 먼저 호출해야 함
button2.loop(); // loop() 함수를 먼저 호출해야 함
button3.loop(); // loop() 함수를 먼저 호출해야 함
button4.loop(); // loop() 함수를 먼저 호출해야 함
button5.loop(); // loop() 함수를 먼저 호출해야 함
// 디바운스 후 버튼 상태 가져오기
int button1_state = button1.getState(); // 디바운스 후 상태
int button2_state = button2.getState(); // 디바운스 후 상태
int button3_state = button3.getState(); // 디바운스 후 상태
int button4_state = button4.getState(); // 디바운스 후 상태
int button5_state = button5.getState(); // 디바운스 후 상태
/*
Serial.print("The button 1 state: ");
Serial.println(button1_state);
Serial.print("The button 2 state: ");
Serial.println(button2_state);
Serial.print("The button 3 state: ");
Serial.println(button3_state);
Serial.print("The button 4 state: ");
Serial.println(button4_state);
Serial.print("The button 5 state: ");
Serial.println(button5_state);
*/
if (button1.isPressed())
Serial.println("The button 1 is pressed");
if (button1.isReleased())
Serial.println("The button 1 is released");
if (button2.isPressed())
Serial.println("The button 2 is pressed");
if (button2.isReleased())
Serial.println("The button 2 is released");
if (button3.isPressed())
Serial.println("The button 3 is pressed");
if (button3.isReleased())
Serial.println("The button 3 is released");
if (button4.isPressed())
Serial.println("The button 4 is pressed");
if (button4.isReleased())
Serial.println("The button 4 is released");
if (button5.isPressed())
Serial.println("The button 5 is pressed");
if (button5.isReleased())
Serial.println("The button 5 is released");
}
사용 방법
- 위 이미지와 같이 전선을 연결하세요.
- Arduino 보드를 USB 케이블을 통해 PC에 연결하세요.
- PC에서 Arduino IDE를 엽니다.
- 올바른 Arduino 보드(예: Arduino Uno)와 COM 포트를 선택하세요.
- Arduino IDE의 왼쪽 바에 있는 라이브러리 아이콘을 클릭하세요.
- "ezButton"을 검색한 다음, ArduinoGetStarted의 버튼 라이브러리를 찾으세요.
- EzButton 라이브러리를 설치하려면 설치 버튼을 클릭하세요.
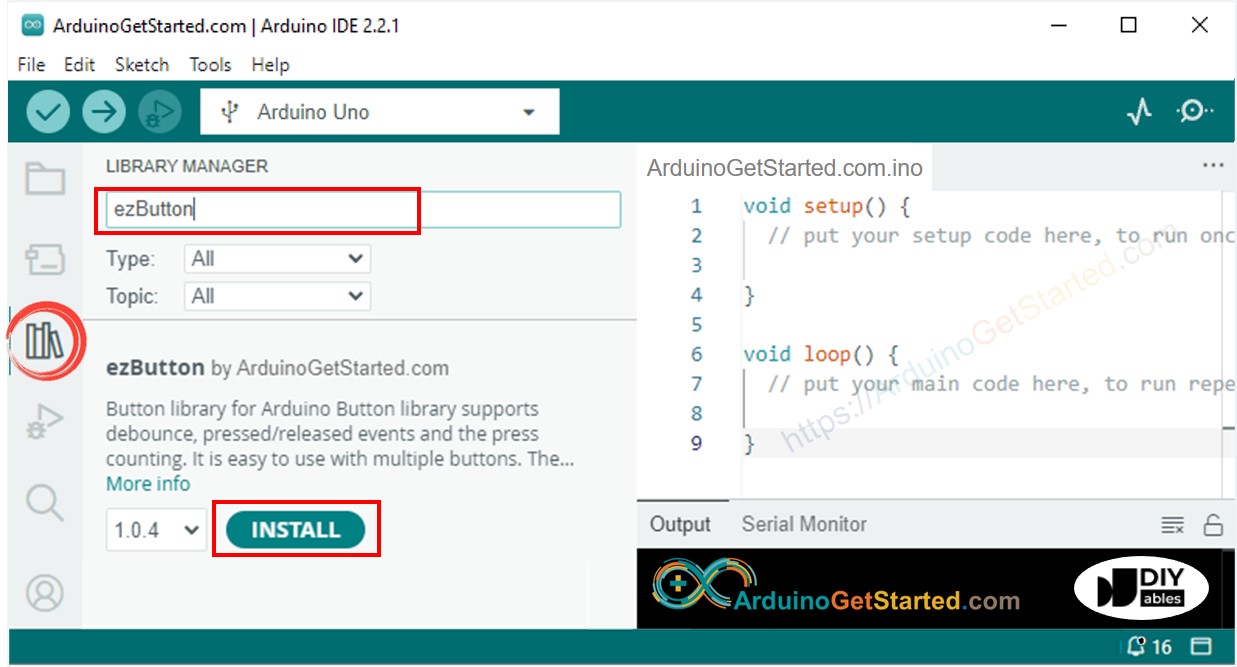
- 위의 코드를 복사하여 아두이노 IDE에 붙여넣으세요.
- 아두이노 IDE에서 Upload 버튼을 클릭하여 코드를 컴파일하고 아두이노 보드에 업로드하세요.
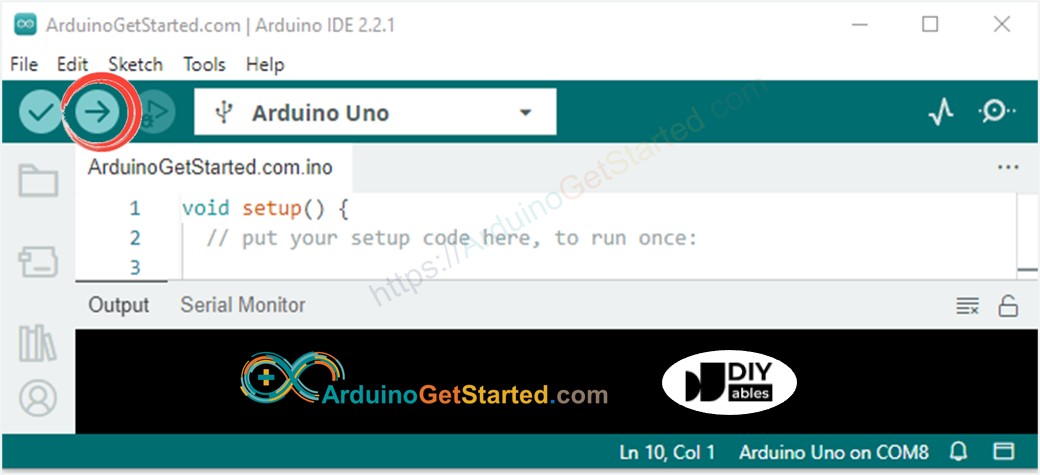
- Arduino IDE에서 시리얼 모니터 열기
- 버튼을 하나씩 눌렀다 놓기
COM6
The button 1 is pressed
The button 1 is released
The button 2 is pressed
The button 2 is released
The button 3 is pressed
The button 3 is released
The button 4 is pressed
The button 4 is released
The button 5 is pressed
The button 5 is released
Autoscroll
Clear output
9600 baud
Newline
아두이노 코드 - 배열을 사용한 다중 버튼
위의 코드를 버튼 배열을 사용하여 개선할 수 있습니다. 다음 코드는 이 배열을 사용하여 버튼 객체를 처리합니다.
/*
* 이 아두이노 코드는 newbiely.kr 에서 개발되었습니다
* 이 아두이노 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-multiple-button
*/
#include <ezButton.h>
#define BUTTON_NUM 5 // 버튼의 수
#define BUTTON_PIN_1 2 // 버튼 1에 연결된 아두이노 핀
#define BUTTON_PIN_2 3 // 버튼 2에 연결된 아두이노 핀
#define BUTTON_PIN_3 4 // 버튼 3에 연결된 아두이노 핀
#define BUTTON_PIN_4 5 // 버튼 4에 연결된 아두이노 핀
#define BUTTON_PIN_5 6 // 버튼 5에 연결된 아두이노 핀
ezButton buttonArray[] = {
ezButton(BUTTON_PIN_1),
ezButton(BUTTON_PIN_2),
ezButton(BUTTON_PIN_3),
ezButton(BUTTON_PIN_4),
ezButton(BUTTON_PIN_5)
};
void setup() {
Serial.begin(9600);
for (byte i = 0; i < BUTTON_NUM; i++) {
buttonArray[i].setDebounceTime(100); // 디바운스 시간을 100밀리초로 설정
}
}
void loop() {
for (byte i = 0; i < BUTTON_NUM; i++)
buttonArray[i].loop(); // loop() 함수를 먼저 호출해야 함
for (byte i = 0; i < BUTTON_NUM; i++) {
// 디바운스 후의 버튼 상태 가져오기
int button_state = buttonArray[i].getState(); // 디바운스 후의 상태
/*
Serial.print("The button ");
Serial.print(i + 1);
Serial.print(": ");
Serial.println(button_state);
*/
if (buttonArray[i].isPressed()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is pressed");
}
if (buttonArray[i].isReleased()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is released");
}
}
}
동영상
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.