아두이노 버튼 디벗싱
버튼이 눌리거나 놓이거나 스위치가 전환될 때, 초보자들은 보통 그 상태가 LOW에서 HIGH로 혹은 HIGH에서 LOW로 바뀐다고 단순하게 생각합니다. 실제로는 그렇지 않습니다. 기계적이고 물리적 특성 때문에 버튼(또는 스위치)의 상태는 여러 번 LOW와 HIGH 사이를 전환할 수 있습니다. 이 현상을 채터링이라고 합니다. 채터링 현상은 단일 누름이 여러 번 눌린 것처럼 읽히게 하여, 어떤 종류의 애플리케이션에서는 오작동을 일으킬 수 있습니다. 이 튜토리얼에서는 이 현상(입력을 디바운스(debounce)한다고 불림)을 없애는 방법을 보여줍니다.
1 | × | 아두이노 우노 R3 | 쿠팡 | 아마존 | |
1 | × | USB 2.0 케이블 타입 A/B | 쿠팡 | 아마존 | |
1 | × | 버튼 키트 | 쿠팡 | 아마존 | |
1 | × | 패널 장착 푸시 버튼 | 아마존 | |
1 | × | 브레드보드 | 쿠팡 | 아마존 | |
1 | × | 점퍼케이블 | 아마존 | |
1 | × | (추천) 아두이노 우노용 스크루 터미널 블록 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 브레드보드 쉴드 | 쿠팡 | 아마존 | |
1 | × | (추천) 아두이노 우노용 케이스 | 쿠팡 | 아마존 | |
공개: 이 섹션에서 제공된 링크 중 일부는 제휴 링크입니다. 이 링크를 통해 구매한 경우 추가 비용없이 수수료를 받을 수 있습니다. 지원해 주셔서 감사합니다.
버튼(핀 배치, 작동 방식, 프로그래밍 방법 등)에 대해 잘 모르신다면, 다음 튜토리얼에서 배워보세요:
아두이노 - 버튼
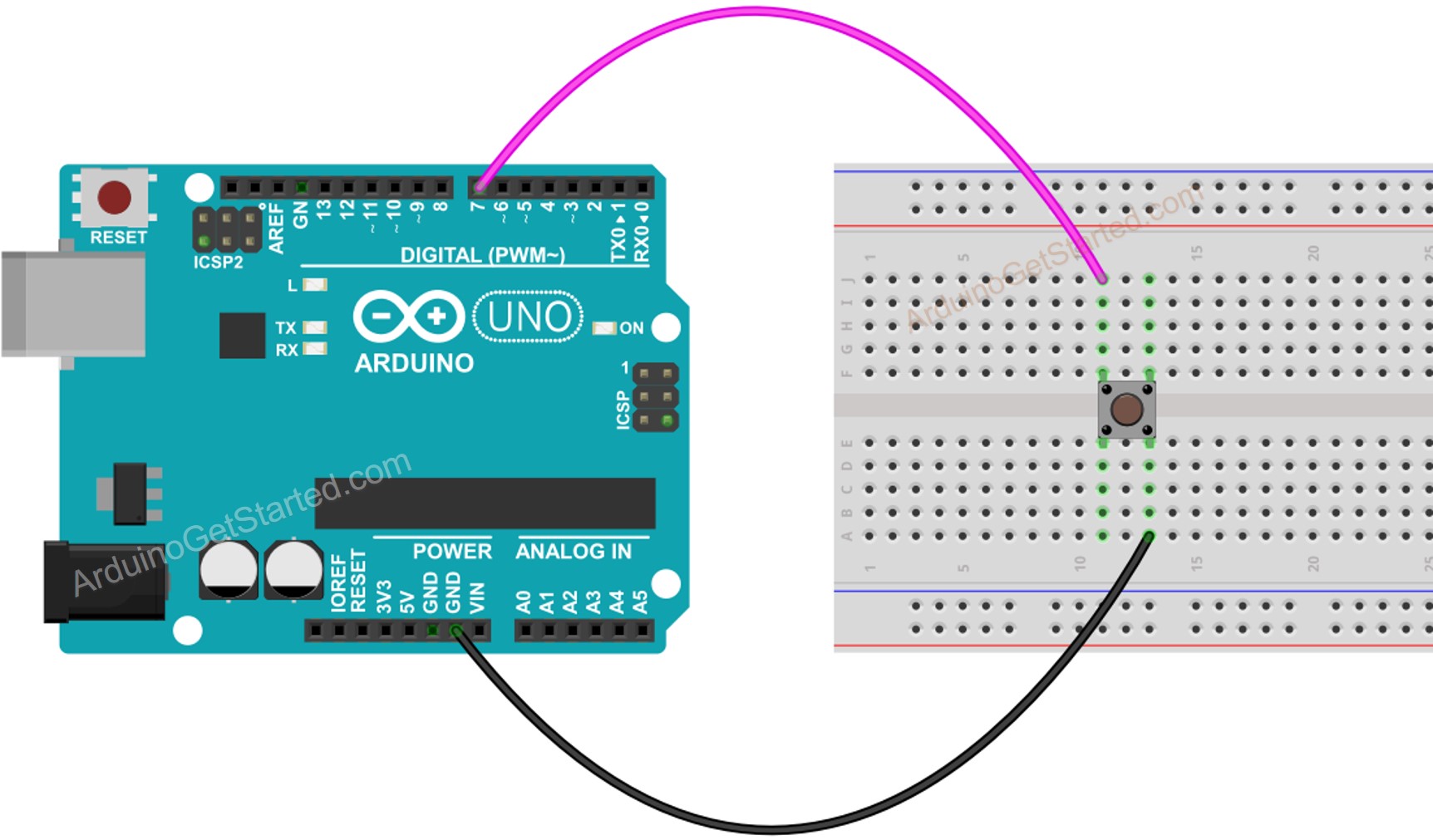
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
WITHOUT 및 WITH debounce와 그들의 동작을 보고 비교해 봅시다.
디바운싱에 대해 배우기 전에, 디바운싱 없이 코드와 그 동작을 먼저 봅시다.
아두이노를 USB 케이블을 통해 PC에 연결하십시오.
아두이노 IDE를 열고, 올바른 보드와 포트를 선택하십시오.
아래 코드를 복사하고 아두이노 IDE로 열십시오.
const int BUTTON_PIN = 7;
int lastState = LOW;
int currentState;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if(lastState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if(lastState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastState = currentState;
}
아두이노 IDE에서 코드를 아두이노에 업로드하려면 Upload 버튼을 클릭하세요.
시리얼 모니터 열기
버튼을 몇 초간 계속 누르고 나서 놓으세요.
시리얼 모니터에서 결과를 확인하세요.
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
⇒ 보시다시피, 단 한 번만 버튼을 누르고 놓았습니다. 그러나 아두이노는 이를 여러 번 누르고 놓은 것으로 인식합니다.
아래 코드를 복사하고 아두이노 IDE로 열기
const int BUTTON_PIN = 7;
const int DEBOUNCE_DELAY = 50;
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int currentState;
unsigned long lastDebounceTime = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
if (currentState != lastFlickerableState) {
lastDebounceTime = millis();
lastFlickerableState = currentState;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_DELAY) {
if (lastSteadyState == HIGH && currentState == LOW)
Serial.println("The button is pressed");
else if (lastSteadyState == LOW && currentState == HIGH)
Serial.println("The button is released");
lastSteadyState = currentState;
}
}
The button is pressed
The button is released
⇒ 보시다시피, 버튼을 단 한 번만 누르고 놓았습니다. 아두이노는 이를 단일 누르기와 놓기로 인식합니다. 따라서 잡음이 제거됩니다.
초보자들, 특히 여러 버튼을 사용할 때 훨씬 더 쉽게 만들기 위해, 우리는 ezButton이라고 부르는 라이브러리를 만들었습니다. 여기서 ezButton 라이브러리에 대해 알아볼 수 있습니다.
#include <ezButton.h>
ezButton button(7);
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
#include <ezButton.h>
ezButton button1(6);
ezButton button2(7);
ezButton button3(8);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(50);
button2.setDebounceTime(50);
button3.setDebounceTime(50);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if(button1.isPressed())
Serial.println("The button 1 is pressed");
if(button1.isReleased())
Serial.println("The button 1 is released");
if(button2.isPressed())
Serial.println("The button 2 is pressed");
if(button2.isReleased())
Serial.println("The button 2 is released");
if(button3.isPressed())
Serial.println("The button 3 is pressed");
if(button3.isReleased())
Serial.println("The button 3 is released");
}
위 코드에 대한 배선도:
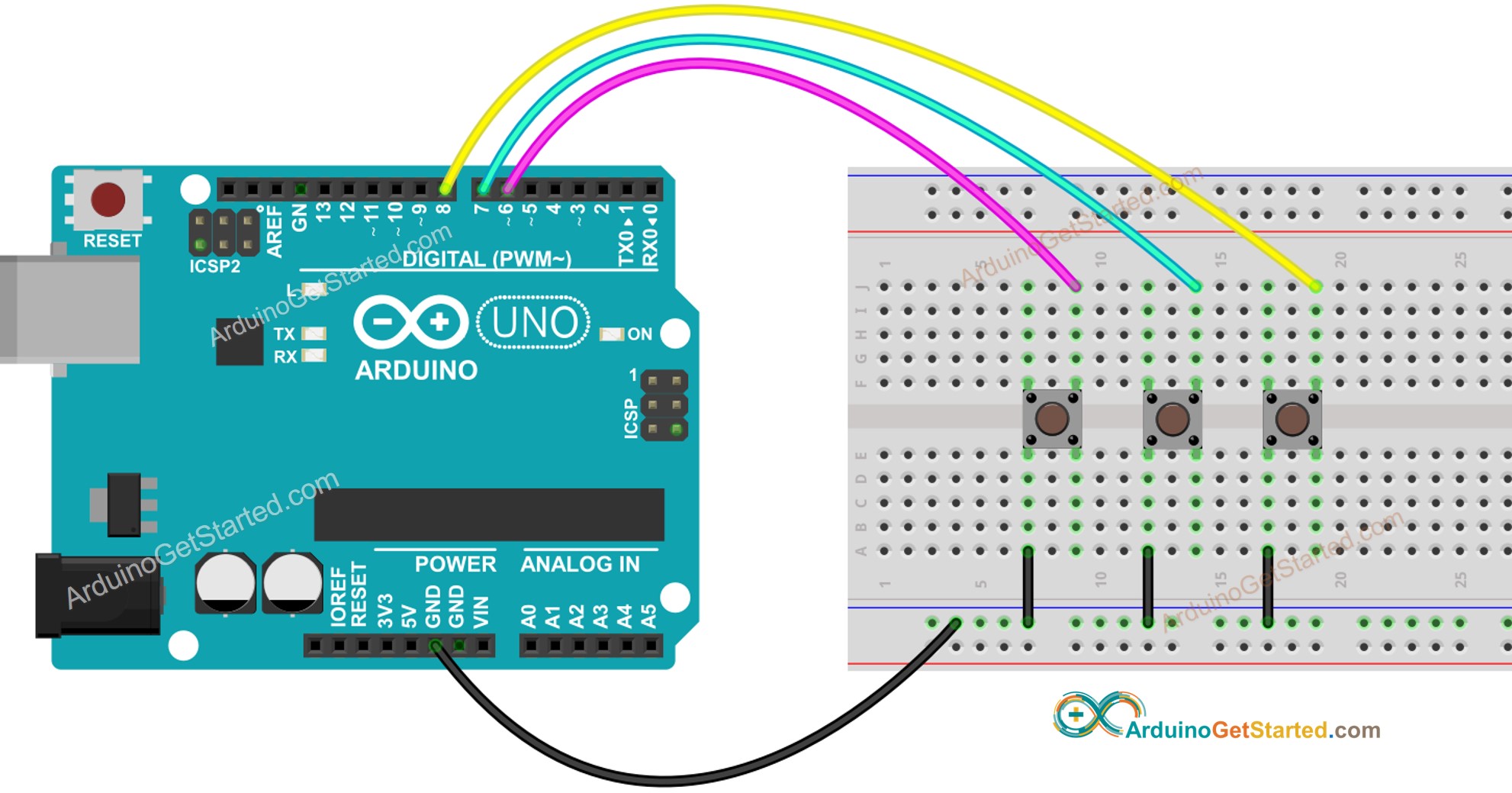
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.
DEBOUNCE_DELAY 값은 응용 프로그램에 따라 다릅니다. 서로 다른 응용 프로그램은 다른 값들을 사용할 수 있습니다.
디바운스 방법은 스위치, 터치 센서 등에 적용할 수 있습니다.