아두이노 - DS1307 RTC 모듈 | Arduino - DS1307 RTC Module
이 튜토리얼에서 우리는 다음을 배우게 됩니다:
- 아두이노와 실시간 시계 DS1307 모듈을 사용하여 데이터 및 시각(초, 분, 시, 일, 날짜, 월, 연도)를 얻는 방법.
- 아두이노로 일정표를 만드는 방법
- 아두이노로 주간 일정표를 만드는 방법
- 아두이노로 특정 날짜에 일정을 만드는 방법
준비물
1 | × | Arduino Uno | Amazon | |
1 | × | USB 2.0 cable type A/B | 쿠팡 | Amazon | |
1 | × | Real-Time Clock DS1307 Module | Amazon | |
1 | × | CR2032 battery | Amazon | |
1 | × | Jumper Wires | Amazon | |
1 | × | (Optional) 9V Power Adapter for Arduino | Amazon | |
1 | × | (Recommended) Screw Terminal Block Shield for Arduino Uno | 쿠팡 | Amazon | |
1 | × | (Recommended) Breadboard Shield For Arduino Uno | 쿠팡 | Amazon | |
1 | × | (Recommended) Enclosure For Arduino Uno | Amazon |
공개: 이 섹션에서 제공된 링크 중 일부는 제휴 링크입니다. 이 링크를 통해 구매한 경우 추가 비용없이 수수료를 받을 수 있습니다. 지원해 주셔서 감사합니다.
실시간 시계 DS1307 모듈에 관하여
아두이노 자체에는 millis(), micros()와 같은 시간 관련 함수가 있습니다. 그러나 이들은 날짜와 시간(초, 분, 시, 일, 날짜, 월, 년)을 제공할 수 없습니다. 날짜와 시간을 얻기 위해서는 DS3231, DS1370과 같은 실시간 시계(RTC) 모듈을 사용해야 합니다. DS3231 모듈은 DS1370보다 더 높은 정밀도를 가집니다. DS3231 vs DS1307을 참조하세요.
핀배열
실시간 시계 DS1307 모듈에는 12개의 핀이 포함되어 있습니다. 그러나 일반적인 사용을 위해서는 4개의 핀을 사용해야 합니다: VCC, GND, SDA, SCL:
- SCL 핀: I2C 인터페이스를 위한 직렬 클록 핀입니다.
- SDA 핀: I2C 인터페이스를 위한 직렬 데이터 핀입니다.
- VCC 핀: 모듈에 전원을 공급합니다. 3.3V에서 5.5V 사이일 수 있습니다.
- GND 핀: 접지 핀입니다.
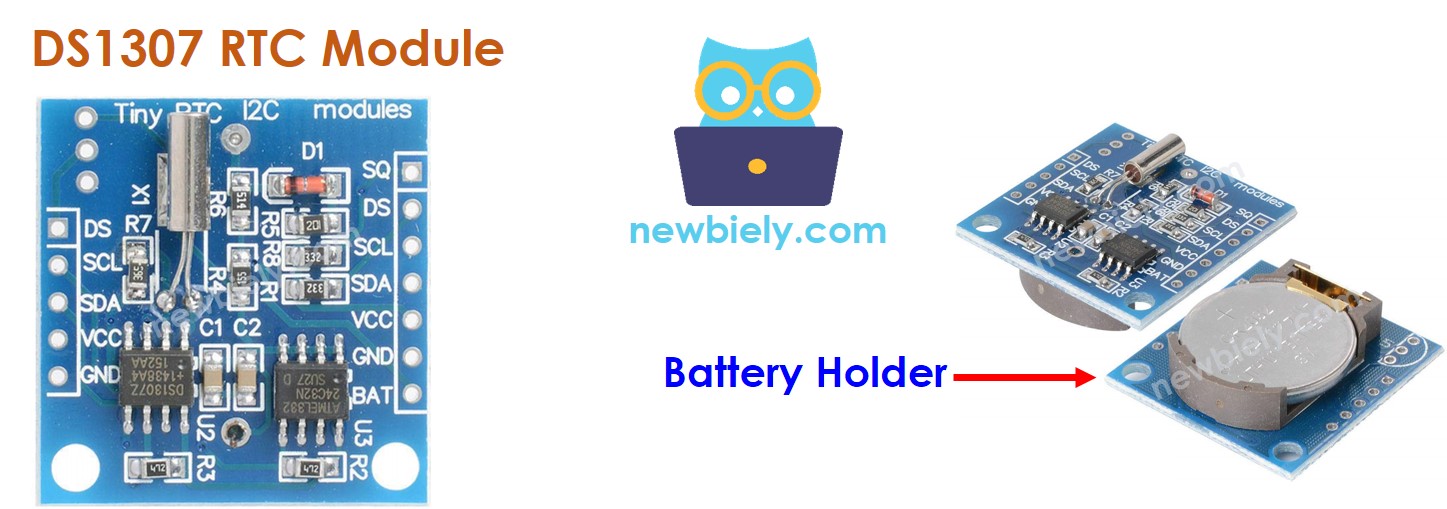
DS1307 모듈에도 배터리 홀더가 있습니다.
- CR2032 배터리를 삽입하면 주 전원이 꺼져 있을 때도 모듈의 시간이 계속 유지됩니다.
- 배터리를 삽입하지 않으면 주 전원이 꺼지면 시간 정보가 사라지고 시간을 다시 설정해야 합니다.
선연결
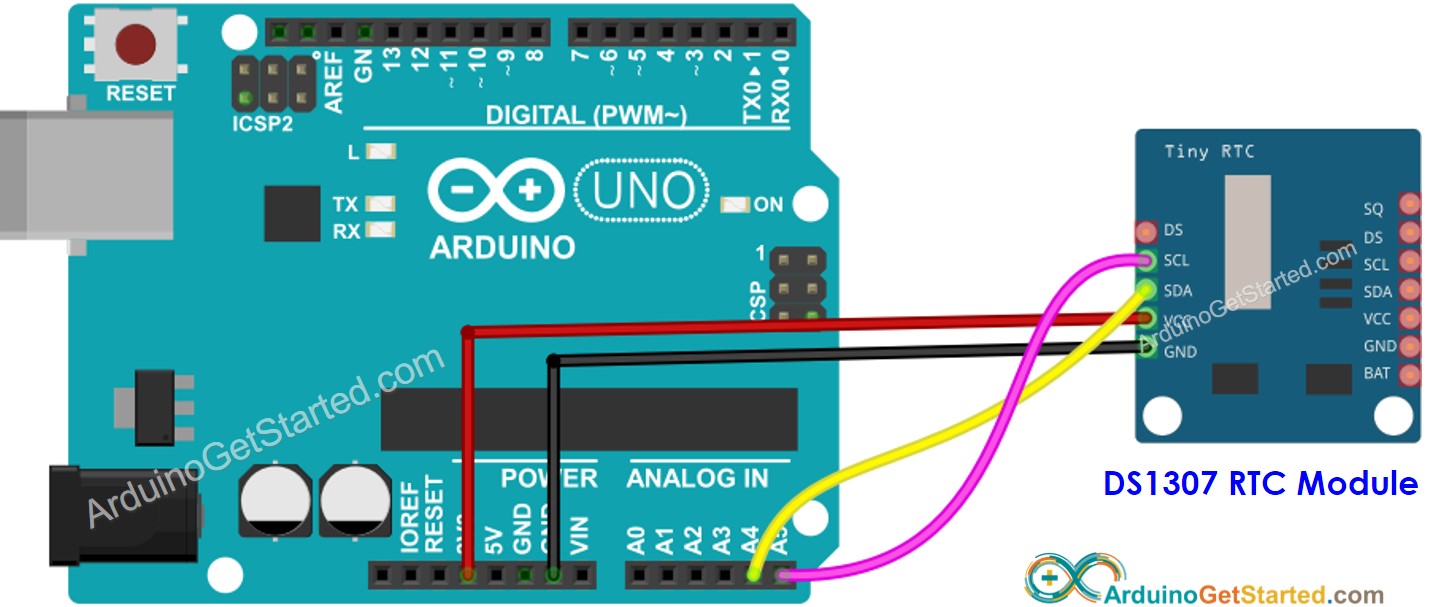
이 이미지는 Fritzing을 사용하여 만들어졌습니다. 이미지를 확대하려면 클릭하세요.
아두이노 - DS1307 RTC 모듈
DS1307 RTC Module | Arduino Uno, Nano | Arduino Mega |
---|---|---|
Vin | 3.3V | 3.3V |
GND | GND | GND |
SDA | A4 | 20 |
SCL | A5 | 21 |
DS1307 RTC 모듈 프로그래밍 방법
라이브러리를 포함하세요:
#include <RTClib.h>
RTC 객체를 선언하십시오:
RTC_DS1307 rtc;
RTC 초기화:
if (! rtc.begin()) {
Serial.println("RTC를 찾을 수 없습니다");
while (1);
}
처음으로, 스케치가 컴파일된 PC의 날짜 및 시간으로 RTC를 설정하세요.
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
RTC 모듈에서 날짜와 시간 정보를 읽습니다.
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(now.dayOfTheWeek());
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
아두이노 코드 - 데이터와 시간을 얻는 방법
/*
* 이 Arduino 코드는 newbiely.kr 에서 개발되었습니다
* 이 Arduino 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-ds1307-rtc-module
*/
#include <RTClib.h>
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
// RTC 모듈 설정
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
// 이 스케치가 컴파일된 PC의 날짜 및 시간으로 RTC를 자동 설정
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// 수동으로 RTC를 명시적인 날짜 및 시간으로 설정, 예를 들어
// 2021년 1월 21일 오전 3시를 설정하려면 다음을 호출하십시오:
// rtc.adjust(DateTime(2021, 1, 21, 3, 0, 0));
}
void loop () {
DateTime now = rtc.now();
Serial.print("Date & Time: ");
Serial.print(now.year(), DEC);
Serial.print('/');
Serial.print(now.month(), DEC);
Serial.print('/');
Serial.print(now.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[now.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(now.hour(), DEC);
Serial.print(':');
Serial.print(now.minute(), DEC);
Serial.print(':');
Serial.println(now.second(), DEC);
delay(1000); // 1초 지연
}
사용 방법
- Arduino IDE의 왼쪽 바에 있는 Libraries 아이콘으로 이동하세요.
- “RTClib”을 검색한 다음, Adafruit의 RTC 라이브러리를 찾으세요.
- RTC 라이브러리를 설치하려면 Install 버튼을 클릭하세요.
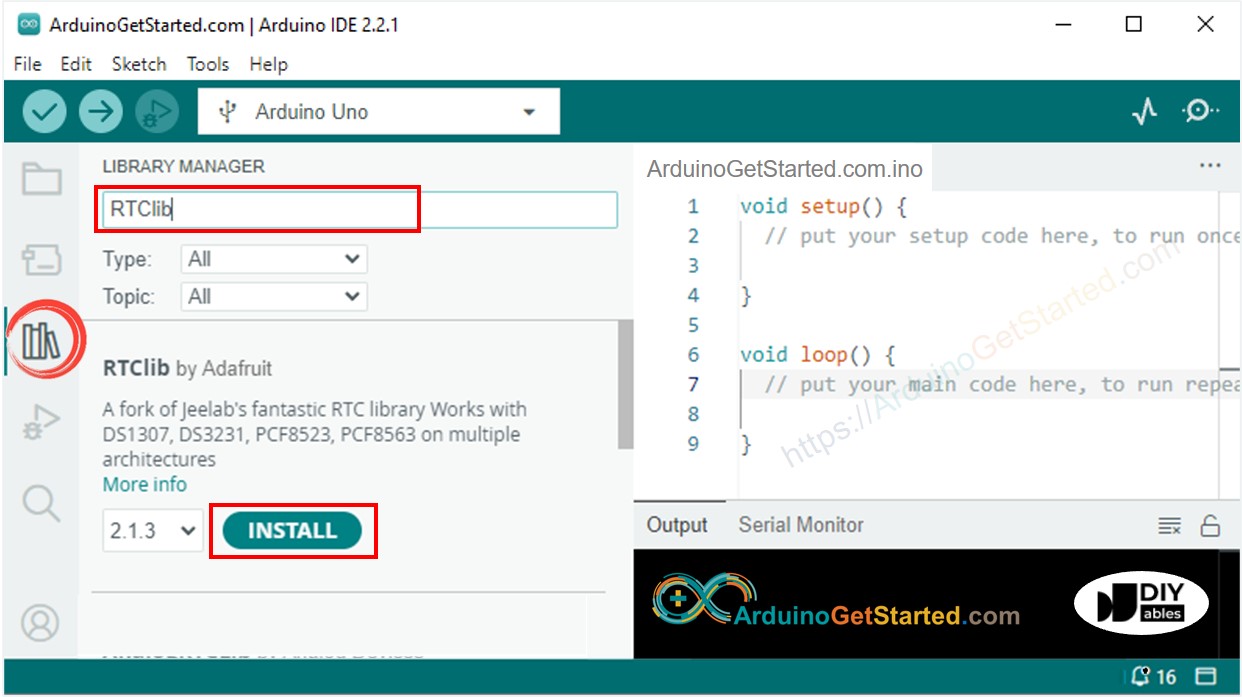
- 다른 라이브러리 의존성을 설치하라는 요청을 받을 수 있습니다
- 모든 라이브러리 의존성을 설치하려면 Install All 버튼을 클릭하세요.
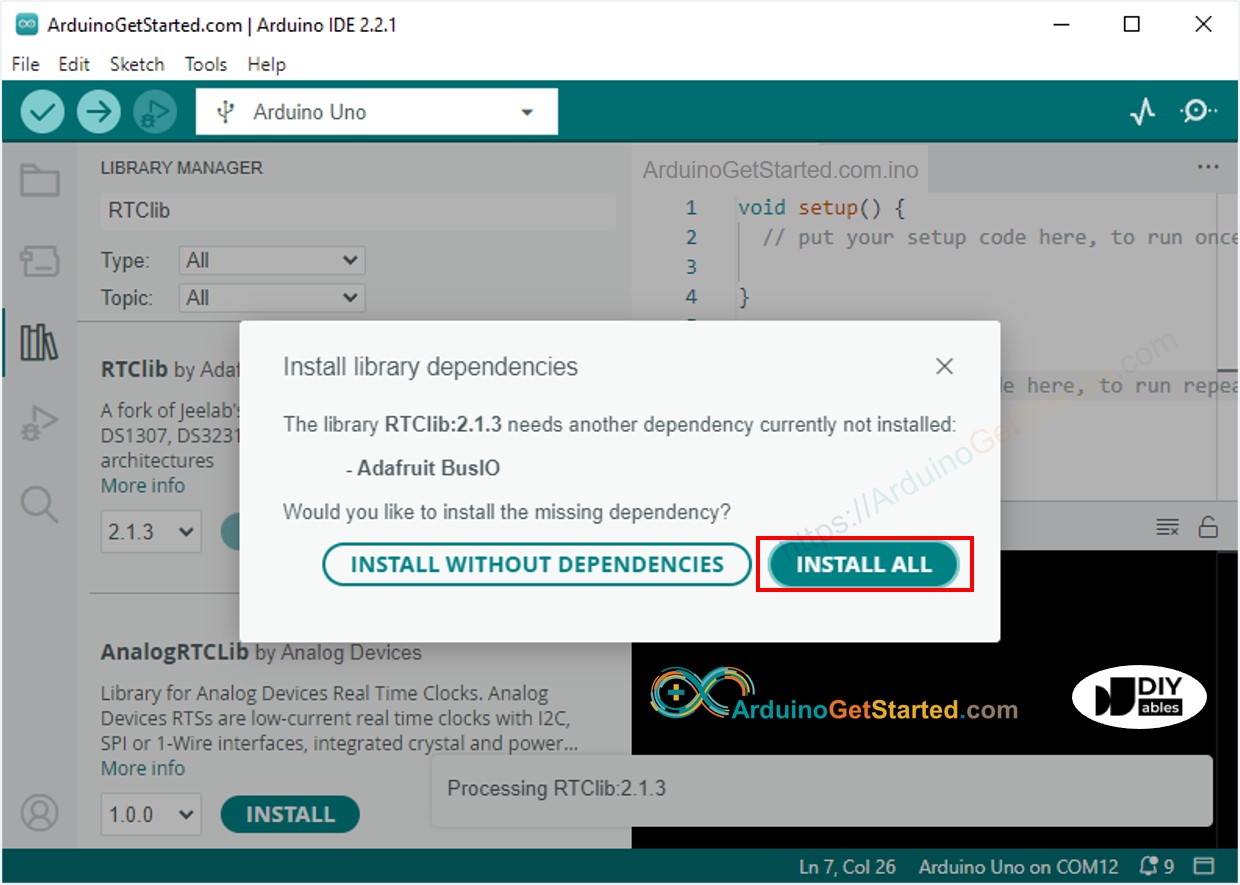
- 위의 코드를 복사하고 Arduino IDE로 열기
- 아두이노 IDE에서 Upload 버튼을 클릭해 아두이노에 코드를 업로드하세요
- 시리얼 모니터 열기
- 시리얼 모니터에서 결과를 확인하세요.
COM6
Date & Time: 2021/10/6 (Wednesday) 9:9:35
Date & Time: 2021/10/6 (Wednesday) 9:9:36
Date & Time: 2021/10/6 (Wednesday) 9:9:37
Date & Time: 2021/10/6 (Wednesday) 9:9:38
Date & Time: 2021/10/6 (Wednesday) 9:9:39
Date & Time: 2021/10/6 (Wednesday) 9:9:40
Date & Time: 2021/10/6 (Wednesday) 9:9:41
Date & Time: 2021/10/6 (Wednesday) 9:9:42
Date & Time: 2021/10/6 (Wednesday) 9:9:43
Date & Time: 2021/10/6 (Wednesday) 9:9:44
Autoscroll
Clear output
9600 baud
Newline
아두이노 코드 - 아두이노로 일일 일정을 만드는 방법
/*
* 이 Arduino 코드는 newbiely.kr 에서 개발되었습니다
* 이 Arduino 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-ds1307-rtc-module
*/
// I2C 및 Wire lib를 통해 연결된 DS1307 RTC를 사용한 날짜 및 시간 기능
#include <RTClib.h>
// 13:50부터 14:10까지의 이벤트
uint8_t DAILY_EVENT_START_HH = 13; // 이벤트 시작 시간: 시
uint8_t DAILY_EVENT_START_MM = 50; // 이벤트 시작 시간: 분
uint8_t DAILY_EVENT_END_HH = 14; // 이벤트 종료 시간: 시
uint8_t DAILY_EVENT_END_MM = 10; // 이벤트 종료 시간: 분
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
// RTC 모듈 설정
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
// 이 스케치가 컴파일된 PC의 날짜 및 시간으로 RTC를 설정
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// 명시적인 날짜 및 시간으로 RTC를 설정, 예를 들어 2021년 1월 21일 오전 3시를 설정하려면 아래와 같이 호출:
// rtc.adjust(DateTime(2021, 1, 21, 3, 0, 0));
}
void loop () {
DateTime now = rtc.now();
if (now.hour() >= DAILY_EVENT_START_HH &&
now.minute() >= DAILY_EVENT_START_MM &&
now.hour() < DAILY_EVENT_END_HH &&
now.minute() < DAILY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
// TODO: 여기에 코드 작성
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
아두이노 코드 - 아두이노로 주간 일정 만드는 방법
/*
* 이 Arduino 코드는 newbiely.kr 에서 개발되었습니다
* 이 Arduino 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-ds1307-rtc-module
*/
// I2C 및 Wire 라이브러리를 통해 연결된 DS1307 RTC를 사용한 날짜와 시간 함수
#include <RTClib.h>
// 변경할 수 없는 매개변수들
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
// 월요일에 일어나는 이벤트, 13:50부터 14:10까지
uint8_t WEEKLY_EVENT_DAY = MONDAY;
uint8_t WEEKLY_EVENT_START_HH = 13; // 이벤트 시작 시간: 시
uint8_t WEEKLY_EVENT_START_MM = 50; // 이벤트 시작 시간: 분
uint8_t WEEKLY_EVENT_END_HH = 14; // 이벤트 종료 시간: 시
uint8_t WEEKLY_EVENT_END_MM = 10; // 이벤트 종료 시간: 분
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
// RTC 모듈 설정
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
while (1);
}
// 이 스케치가 컴파일된 PC의 날짜 및 시간으로 RTC를 설정
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// 명시적인 날짜 및 시간으로 RTC를 설정하는 경우, 예를 들어
// 2021년 1월 21일 오전 3시를 설정하려면 다음과 같이 호출:
// rtc.adjust(DateTime(2021, 1, 21, 3, 0, 0));
}
void loop () {
DateTime now = rtc.now();
if (now.dayOfTheWeek() == WEEKLY_EVENT_DAY &&
now.hour() >= WEEKLY_EVENT_START_HH &&
now.minute() >= WEEKLY_EVENT_START_MM &&
now.hour() < WEEKLY_EVENT_END_HH &&
now.minute() < WEEKLY_EVENT_END_MM) {
Serial.println("It is on scheduled time");
// 할 일: 여기에 코드를 작성하세요
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
아두이노 코드 - 특정 날짜에 일정을 만드는 방법
/*
* 이 Arduino 코드는 newbiely.kr 에서 개발되었습니다
* 이 Arduino 코드는 어떠한 제한 없이 공개 사용을 위해 제공됩니다.
* 상세한 지침 및 연결도에 대해서는 다음을 방문하세요:
* https://newbiely.kr/tutorials/arduino/arduino-ds1307-rtc-module
*/
// DS1307 RTC를 I2C와 Wire lib를 사용하여 날짜 및 시간 기능
#include <RTClib.h>
// 변경할 수 없는 매개변수들
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
#define JANUARY 1
#define FEBRUARY 2
#define MARCH 3
#define APRIL 4
#define MAY 5
#define JUNE 6
#define JULY 7
#define AUGUST 8
#define SEPTEMBER 9
#define OCTOBER 10
#define NOVEMBER 11
#define DECEMBER 12
// 2021년 8월 15일 13:50부터 2021년 9월 29일 14:10까지의 이벤트
DateTime EVENT_START(2021, AUGUST, 15, 13, 50);
DateTime EVENT_END(2021, SEPTEMBER, 29, 14, 10);
RTC_DS1307 rtc;
char daysOfTheWeek[7][12] = {
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday"
};
void setup () {
Serial.begin(9600);
// RTC 모듈 설정
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (1);
}
// 이 스케치가 컴파일된 PC의 날짜 및 시간으로 RTC를 설정
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
// 명시적인 날짜와 시간으로 RTC를 설정, 예를 들어
// 2021년 1월 21일 오전 3시를 설정하려면 다음을 호출하면 됩니다:
// rtc.adjust(DateTime(2021, 1, 21, 3, 0, 0));
}
void loop () {
DateTime now = rtc.now();
if (now.secondstime() >= EVENT_START.secondstime() &&
now.secondstime() < EVENT_END.secondstime()) {
Serial.println("It is on scheduled time");
// TODO: write your code"
} else {
Serial.println("It is NOT on scheduled time");
}
printTime(now);
}
void printTime(DateTime time) {
Serial.print("TIME: ");
Serial.print(time.year(), DEC);
Serial.print('/');
Serial.print(time.month(), DEC);
Serial.print('/');
Serial.print(time.day(), DEC);
Serial.print(" (");
Serial.print(daysOfTheWeek[time.dayOfTheWeek()]);
Serial.print(") ");
Serial.print(time.hour(), DEC);
Serial.print(':');
Serial.print(time.minute(), DEC);
Serial.print(':');
Serial.println(time.second(), DEC);
}
동영상
비디오 제작은 시간이 많이 걸리는 작업입니다. 비디오 튜토리얼이 학습에 도움이 되었다면, YouTube 채널 을 구독하여 알려 주시기 바랍니다. 비디오에 대한 높은 수요가 있다면, 비디오를 만들기 위해 노력하겠습니다.
댓글
WARNING
이 튜토리얼은 개발 중입니다.